用go语言写一个可以存储学生所有信息,不会覆盖学生上一条信息的智能合约
时间: 2024-06-12 08:08:06 浏览: 52
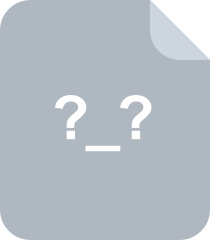
存储学校学生信息C++编写

package main
import (
"encoding/json"
"fmt"
"github.com/hyperledger/fabric/core/chaincode/shim"
"github.com/hyperledger/fabric/protos/peer"
)
type Student struct {
ID string `json:"id"`
Name string `json:"name"`
Age int `json:"age"`
Gender string `json:"gender"`
Grade string `json:"grade"`
Previous string `json:"previous"`
}
type StudentChaincode struct {
}
func (s *StudentChaincode) Init(stub shim.ChaincodeStubInterface) peer.Response {
return shim.Success(nil)
}
func (s *StudentChaincode) Invoke(stub shim.ChaincodeStubInterface) peer.Response {
function, args := stub.GetFunctionAndParameters()
switch function {
case "addStudent":
return s.addStudent(stub, args)
case "getStudent":
return s.getStudent(stub, args)
default:
return shim.Error("Invalid function name")
}
}
func (s *StudentChaincode) addStudent(stub shim.ChaincodeStubInterface, args []string) peer.Response {
if len(args) != 5 {
return shim.Error("Incorrect number of arguments. Expecting 5")
}
id := args[0]
name := args[1]
age := args[2]
gender := args[3]
grade := args[4]
student := &Student{
ID: id,
Name: name,
Age: age,
Gender: gender,
Grade: grade,
Previous: "",
}
studentBytes, err := json.Marshal(student)
if err != nil {
return shim.Error("Failed to marshal student")
}
err = stub.PutState(id, studentBytes)
if err != nil {
return shim.Error(fmt.Sprintf("Failed to add student to ledger: %s", err.Error()))
}
return shim.Success(nil)
}
func (s *StudentChaincode) getStudent(stub shim.ChaincodeStubInterface, args []string) peer.Response {
if len(args) != 1 {
return shim.Error("Incorrect number of arguments. Expecting student ID")
}
id := args[0]
studentBytes, err := stub.GetState(id)
if err != nil {
return shim.Error(fmt.Sprintf("Failed to get student from ledger: %s", err.Error()))
}
if studentBytes == nil {
return shim.Error(fmt.Sprintf("Student with ID %s does not exist", id))
}
var student Student
err = json.Unmarshal(studentBytes, &student)
if err != nil {
return shim.Error("Failed to unmarshal student")
}
if student.Previous != "" {
previousStudentBytes, err := stub.GetState(student.Previous)
if err != nil {
return shim.Error(fmt.Sprintf("Failed to get previous student from ledger: %s", err.Error()))
}
var previousStudent Student
err = json.Unmarshal(previousStudentBytes, &previousStudent)
if err != nil {
return shim.Error("Failed to unmarshal previous student")
}
student.Previous = string(previousStudentBytes)
}
return shim.Success(studentBytes)
}
func main() {
err := shim.Start(new(StudentChaincode))
if err != nil {
fmt.Printf("Error starting StudentChaincode: %s", err)
}
}
阅读全文
相关推荐
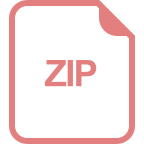
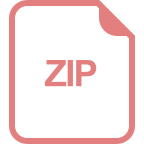
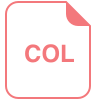
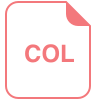
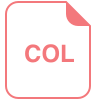
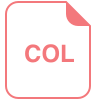
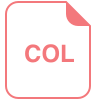
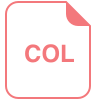
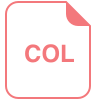
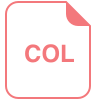
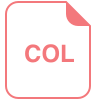
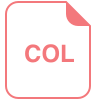
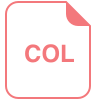
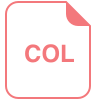
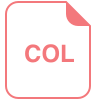
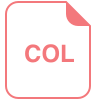

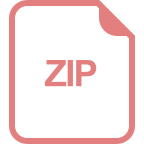