高斯过程GPC 分类器 C++代码
时间: 2023-09-05 17:13:57 浏览: 52
以下是一个简单的高斯过程分类器(GPC)的 C++ 代码示例:
```c++
#include <iostream>
#include <Eigen/Dense>
#include <Eigen/Cholesky>
#include <cmath>
using namespace Eigen;
class GaussianProcessClassifier {
private:
MatrixXd X_train; // 训练数据
VectorXd y_train; // 目标值
double sigma_f; // 高斯过程的振幅
double sigma_n; // 噪声的标准差
double l; // 高斯过程的长度尺度
MatrixXd K_inv; // 训练数据的协方差矩阵的逆矩阵
public:
GaussianProcessClassifier(MatrixXd X, VectorXd y, double sf, double sn, double length) {
X_train = X;
y_train = y;
sigma_f = sf;
sigma_n = sn;
l = length;
int n = X_train.rows(); // 训练数据的数量
MatrixXd K(n, n); // 协方差矩阵
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
double dist = (X_train.row(i) - X_train.row(j)).norm();
K(i, j) = sigma_f * exp(-0.5 * pow(dist / l, 2));
if (i == j) {
K(i, j) += pow(sigma_n, 2);
}
}
}
K_inv = K.inverse();
}
double predict(VectorXd x) {
int n = X_train.rows(); // 训练数据的数量
VectorXd k(n); // 预测点和训练数据之间的协方差向量
for (int i = 0; i < n; i++) {
double dist = (X_train.row(i) - x.transpose()).norm();
k(i) = sigma_f * exp(-0.5 * pow(dist / l, 2));
}
VectorXd mu = k.transpose() * K_inv * y_train; // 预测点的均值
VectorXd sigma = sigma_f - k.transpose() * K_inv * k; // 预测点的标准差
double prob = 1.0 / (1.0 + exp(-mu(0) / sqrt(1.0 + pow(M_PI * sigma(0), 2) / 8.0))); // 通过 probit 函数转化为概率
return prob;
}
};
int main() {
MatrixXd X_train(3, 2);
VectorXd y_train(3);
X_train << 1.0, 2.0,
3.0, 4.0,
5.0, 6.0;
y_train << 1.0,
-1.0,
1.0;
GaussianProcessClassifier gpc(X_train, y_train, 1.0, 0.1, 1.0);
VectorXd x(2);
x << 2.5, 4.5;
std::cout << "预测点的概率是:" << gpc.predict(x) << std::endl;
return 0;
}
```
这个 GPC 的实现使用了 Eigen 库来进行矩阵和向量计算。在构造函数中,我们首先计算了训练数据的协方差矩阵的逆矩阵。在预测函数中,我们首先计算了预测点和训练数据之间的协方差向量,然后通过协方差向量和训练数据的协方差矩阵的逆矩阵来计算预测点的均值和标准差。最后,我们通过 probit 函数将均值和标准差转化为概率。
相关推荐
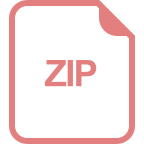
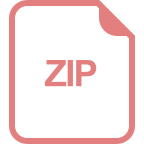














