python对文本预处理代码
时间: 2023-10-17 17:24:30 浏览: 114
以下是 Python 中常用的文本预处理代码:
1. 分词:
使用第三方库 `jieba` 进行中文分词:
```python
import jieba
# 将文本进行分词
text = "你好,我是一个AI助手。"
seg_list = jieba.cut(text, cut_all=False)
print(" ".join(seg_list)) # 输出分词结果,用空格隔开
```
2. 去除停用词:
使用第三方库 `nltk` 进行英文停用词的去除:
```python
import nltk
from nltk.corpus import stopwords
# 下载停用词表
nltk.download('stopwords')
# 定义停用词列表
stop_words = stopwords.words('english')
# 将文本进行分词
text = "This is a sample sentence, showing off the stop words filtration."
words = text.lower().split()
# 去除停用词
filtered_words = [word for word in words if word not in stop_words]
print(filtered_words)
```
使用第三方库 `jieba` 进行中文停用词的去除:
```python
import jieba
# 定义停用词列表
stop_words = ['的', '了', '是', '我', '你', '他', '她']
# 将文本进行分词
text = "你好,我是一个AI助手。"
seg_list = jieba.cut(text, cut_all=False)
# 去除停用词
filtered_words = [word for word in seg_list if word not in stop_words]
print(" ".join(filtered_words)) # 输出去除停用词后的分词结果,用空格隔开
```
3. 去除标点符号:
使用 Python 内置的 `string` 模块进行标点符号的去除:
```python
import string
# 定义标点符号列表
punctuation = string.punctuation
# 将文本进行分词
text = "This is a sample sentence, showing off the punctuation removal."
# 去除标点符号
filtered_text = "".join([char for char in text if char not in punctuation])
print(filtered_text)
```
使用第三方库 `jieba` 进行中文标点符号的去除:
```python
import jieba
# 定义标点符号列表
punctuation = '!?。"#$%&'()*+,-/:;<=>@[\]^_`{|}~、。,《》【】'
# 将文本进行分词
text = "你好,我是一个AI助手。"
seg_list = jieba.cut(text, cut_all=False)
# 去除标点符号
filtered_words = [word for word in seg_list if word not in punctuation]
print(" ".join(filtered_words)) # 输出去除标点符号后的分词结果,用空格隔开
```
4. 文本表示:
使用第三方库 `sklearn` 进行文本向量化:
```python
from sklearn.feature_extraction.text import CountVectorizer
# 定义文本列表
texts = ["This is a sample sentence.", "Another sample sentence.", "I love Python!"]
# 创建 CountVectorizer 对象
vectorizer = CountVectorizer()
# 对文本进行向量化
vectorized_texts = vectorizer.fit_transform(texts)
# 输出向量化后的结果
print(vectorized_texts.toarray())
```
以上是一些常用的文本预处理代码,不同的文本预处理任务可能需要使用不同的代码。
阅读全文
相关推荐
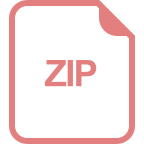
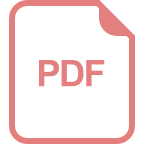
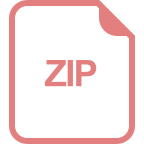
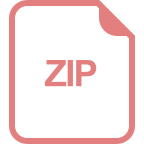
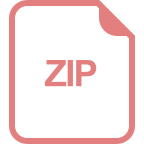
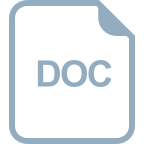
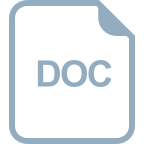
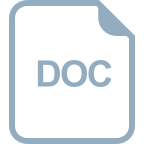
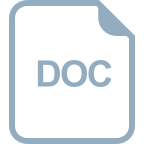
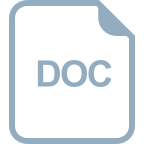
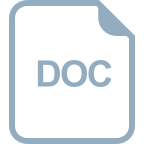
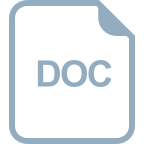

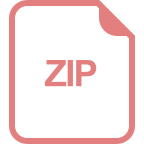
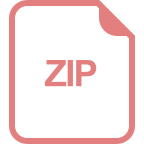