编写stm32f103c8t6利用AD采集电压信号并通过串口输出的程序,使用标准库,PA0为输入接口
时间: 2023-12-06 09:44:16 浏览: 55
下面是基于标准库的 STM32F103C8T6 采集 ADC 电压并通过串口输出的程序。
```c
#include "stm32f10x.h"
#include "stdio.h"
#define ADC1_DR_Address ((u32)0x4001244C)
// 定义 ADC 初始化函数
void ADC1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
ADC_InitTypeDef ADC_InitStructure;
DMA_InitTypeDef DMA_InitStructure;
// 使能 ADC1,GPIOA,DMA1 时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1 | RCC_APB2Periph_GPIOA | RCC_AHBPeriph_DMA1, ENABLE);
// 配置 GPIOA.0 为模拟输入口
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AIN;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// ADC1 工作模式配置
ADC_InitStructure.ADC_Mode = ADC_Mode_Independent; // 独立模式
ADC_InitStructure.ADC_ScanConvMode = DISABLE; // 单通道模式
ADC_InitStructure.ADC_ContinuousConvMode = DISABLE; // 单次转换模式
ADC_InitStructure.ADC_ExternalTrigConv = ADC_ExternalTrigConv_None; // 不使用外部触发
ADC_InitStructure.ADC_DataAlign = ADC_DataAlign_Right; // 数据右对齐
ADC_InitStructure.ADC_NbrOfChannel = 1; // 转换通道数目为1
ADC_Init(ADC1, &ADC_InitStructure); // 初始化 ADC1
// 配置 DMA 通道
DMA_DeInit(DMA1_Channel1); // 复位 DMA1 的通道1
DMA_InitStructure.DMA_PeripheralBaseAddr = ADC1_DR_Address; // ADC1 数据寄存器地址
DMA_InitStructure.DMA_MemoryBaseAddr = (u32)&ADC_ConvertedValue; // DMA 传输数据的内存地址
DMA_InitStructure.DMA_DIR = DMA_DIR_PeripheralSRC; // 数据传输方向,从外设到内存
DMA_InitStructure.DMA_BufferSize = 1; // DMA 缓存大小
DMA_InitStructure.DMA_PeripheralInc = DMA_PeripheralInc_Disable; // 禁止外设地址增量
DMA_InitStructure.DMA_MemoryInc = DMA_MemoryInc_Disable; // 禁止内存地址增量
DMA_InitStructure.DMA_PeripheralDataSize = DMA_PeripheralDataSize_HalfWord; // 外设数据宽度为半字(16位)
DMA_InitStructure.DMA_MemoryDataSize = DMA_MemoryDataSize_HalfWord; // 内存数据宽度为半字(16位)
DMA_InitStructure.DMA_Mode = DMA_Mode_Circular; // 循环传输模式
DMA_InitStructure.DMA_Priority = DMA_Priority_High; // DMA 优先级为高
DMA_InitStructure.DMA_M2M = DMA_M2M_Disable; // 禁止内存到内存传输
DMA_Init(DMA1_Channel1, &DMA_InitStructure); // 初始化 DMA1 的通道1
// 启动 DMA 通道
DMA_Cmd(DMA1_Channel1, ENABLE);
// 开启 ADC1 的 DMA 传输
ADC_DMACmd(ADC1, ENABLE);
// 启动 ADC1 的软件转换
ADC_SoftwareStartConvCmd(ADC1, ENABLE);
}
// 定义 USART 初始化函数
void USART1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
// 使能 USART1 和 GPIOA 时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
// 配置 USART1 的 Tx 引脚为推挽复用模式
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置 USART1 的 Rx 引脚为浮空输入模式
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// USART1 工作模式配置
USART_InitStructure.USART_BaudRate = 115200; // 波特率为115200
USART_InitStructure.USART_WordLength = USART_WordLength_8b; // 数据位为8位
USART_InitStructure.USART_StopBits = USART_StopBits_1; // 停止位为1位
USART_InitStructure.USART_Parity = USART_Parity_No; // 无奇偶校验
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None; // 无硬件流控制
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx; // 收发模式
USART_Init(USART1, &USART_InitStructure); // 初始化 USART1
// 启动 USART1
USART_Cmd(USART1, ENABLE);
}
// 定义格式化输出函数
int fputc(int ch, FILE *f)
{
USART_SendData(USART1, (u8)ch);
while (USART_GetFlagStatus(USART1, USART_FLAG_TC) == RESET);
return ch;
}
u16 ADC_ConvertedValue; // 定义 ADC 转换结果变量
int main(void)
{
ADC1_Init(); // 初始化 ADC1
USART1_Init(); // 初始化 USART1
while (1)
{
printf("ADC value: %d\r\n", ADC_ConvertedValue); // 通过串口输出 ADC 转换结果
delay_ms(500); // 延时 500ms
}
}
```
在上述代码中,我们使用了 `ADC1_Init()` 函数来初始化 ADC1,该函数将 PA0 配置为模拟输入口,并启用了 DMA 传输,以便将 ADC 的转换结果传输到内存中。然后,我们使用了 `USART1_Init()` 函数来初始化 USART1,该函数将 PA9 配置为 USART1 的 Tx 引脚,并将波特率设置为 115200。最后,在 `main()` 函数中,我们使用了 `printf()` 函数将 ADC 转换结果输出到串口。需要注意的是,在输出前需要使用 `delay_ms()` 函数延时一段时间,否则串口会输出过快导致数据无法正常接收。
相关推荐
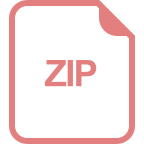
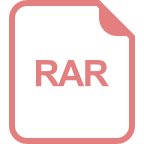
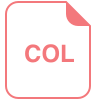
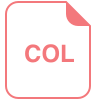
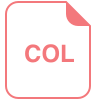
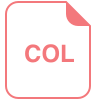
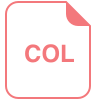



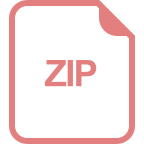
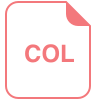
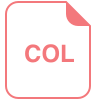
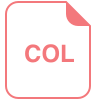
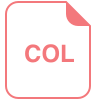