Flask vue 开发网页
时间: 2023-06-12 09:06:52 浏览: 133
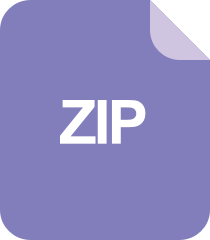
Flask Web开发
Flask 和 Vue 都是非常流行的 Web 开发框架。Flask 是一个用 Python 编写的轻量级 Web 框架,它提供了简单易用的路由、ORM、模板引擎等功能,可以用来快速搭建 Web 应用程序。Vue 是一个用于构建用户界面的渐进式框架,它可以与各种后端框架集成,提供了简单易用的数据绑定、组件化、路由等功能。
下面是一个使用 Flask 和 Vue 开发网页的简单示例:
1. 创建一个 Flask 应用程序
```python
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
```
2. 创建一个 Vue 组件
```vue
<template>
<div>
<h1>{{ message }}</h1>
</div>
</template>
<script>
export default {
data() {
return {
message: 'Hello, World!'
}
}
}
</script>
```
3. 在 Flask 应用程序中引入 Vue 组件
```python
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
```
4. 在 index.html 中加载 Vue 组件
```html
<!DOCTYPE html>
<html>
<head>
<title>Flask Vue App</title>
</head>
<body>
<div id="app">
<my-component></my-component>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script src="{{ url_for('static', filename='js/app.js') }}"></script>
</body>
</html>
```
5. 在 app.js 中注册 Vue 组件
```javascript
import Vue from 'vue'
import MyComponent from './components/MyComponent.vue'
Vue.component('my-component', MyComponent)
new Vue({
el: '#app'
})
```
6. 运行 Flask 应用程序和 Vue 开发服务器
```bash
# 运行 Flask 应用程序
$ python app.py
# 运行 Vue 开发服务器
$ cd static
$ npm install
$ npm run serve
```
这样就可以在浏览器中访问 http://localhost:5000 查看网页了。
阅读全文
相关推荐
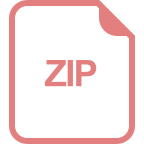
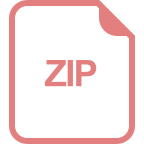
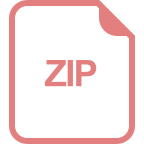
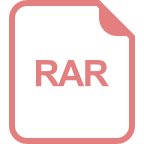
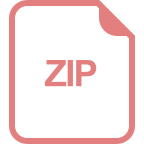
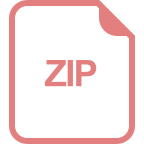
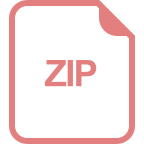
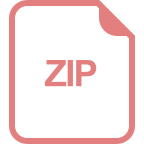
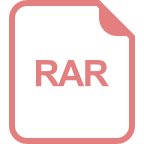
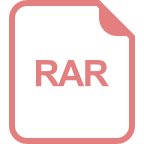
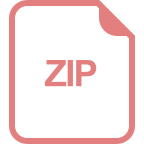
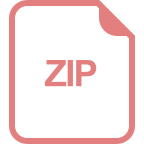
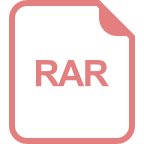
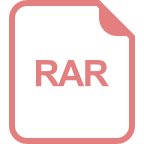
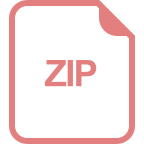
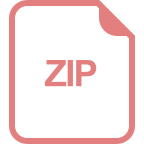