用C语言写一个清空空文件夹的代码
时间: 2024-06-12 22:06:45 浏览: 12
以下是一个清空空文件夹的C语言代码,但请注意,该代码只能清空空文件夹,不会删除非空文件夹中的文件。
```c
#include <stdio.h>
#include <stdlib.h>
#include <dirent.h>
#include <string.h>
void empty_directory(char *path);
int main() {
char path[100];
printf("Enter the path of the directory to empty: ");
scanf("%s", path);
empty_directory(path);
printf("Directory %s has been emptied.\n", path);
return 0;
}
void empty_directory(char *path) {
struct dirent *entry;
DIR *dir = opendir(path);
char filepath[100];
if (dir == NULL) {
printf("Unable to open directory: %s\n", path);
return;
}
while ((entry = readdir(dir)) != NULL) {
if (strcmp(entry->d_name, ".") != 0 && strcmp(entry->d_name, "..") != 0) {
sprintf(filepath, "%s/%s", path, entry->d_name);
if (entry->d_type == DT_DIR) {
empty_directory(filepath);
rmdir(filepath);
} else {
remove(filepath);
}
}
}
closedir(dir);
}
```
在该代码中,我们首先定义了一个 `empty_directory` 函数,该函数接受一个文件夹路径作为参数。该函数会打开该文件夹并读取其中的所有文件和子文件夹,如果遇到子文件夹,我们将递归调用 `empty_directory` 函数来清空该子文件夹。如果遇到文件,我们直接使用 `remove` 函数将其删除。最后,我们使用 `rmdir` 函数删除空文件夹。
在 `main` 函数中,我们首先要求用户输入要清空的文件夹路径,然后调用 `empty_directory` 函数来清空该文件夹。
相关推荐
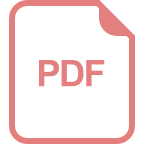
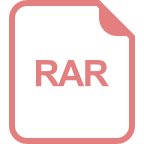
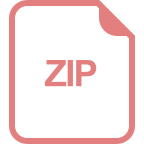














