如何用Python制作一个交通灯控制小游戏
时间: 2024-04-29 22:23:01 浏览: 168
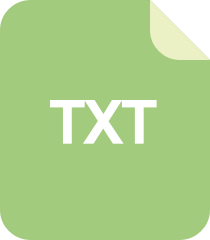
小型交通灯控制的程序
以下是一个简单的交通灯控制小游戏的Python代码示例:
```python
import tkinter as tk
class TrafficLightGame(tk.Frame):
def __init__(self, master=None):
tk.Frame.__init__(self, master)
self.grid()
self.create_widgets()
def create_widgets(self):
self.red_on = tk.PhotoImage(file="red_on.png")
self.red_off = tk.PhotoImage(file="red_off.png")
self.yellow_on = tk.PhotoImage(file="yellow_on.png")
self.yellow_off = tk.PhotoImage(file="yellow_off.png")
self.green_on = tk.PhotoImage(file="green_on.png")
self.green_off = tk.PhotoImage(file="green_off.png")
self.red_light = tk.Label(self, image=self.red_on)
self.red_light.grid(row=0, column=0, padx=5, pady=5)
self.yellow_light = tk.Label(self, image=self.yellow_off)
self.yellow_light.grid(row=1, column=0, padx=5, pady=5)
self.green_light = tk.Label(self, image=self.green_off)
self.green_light.grid(row=2, column=0, padx=5, pady=5)
self.start_button = tk.Button(self, text="Start", command=self.start_game)
self.start_button.grid(row=3, column=0, padx=5, pady=5)
def start_game(self):
self.start_button.config(state=tk.DISABLED)
self.red_light.config(image=self.red_on)
self.yellow_light.config(image=self.yellow_off)
self.green_light.config(image=self.green_off)
self.after(5000, self.change_yellow)
def change_yellow(self):
self.red_light.config(image=self.red_off)
self.yellow_light.config(image=self.yellow_on)
self.green_light.config(image=self.green_off)
self.after(2000, self.change_green)
def change_green(self):
self.red_light.config(image=self.red_off)
self.yellow_light.config(image=self.yellow_off)
self.green_light.config(image=self.green_on)
self.after(5000, self.change_yellow)
game = TrafficLightGame()
game.master.title("Traffic Light Game")
game.mainloop()
```
代码中使用了tkinter模块来创建GUI界面。游戏界面上有三个交通灯标志,分别表示红灯、黄灯和绿灯。开始游戏后,红灯亮起5秒钟,然后黄灯亮起2秒钟,接着绿灯亮起5秒钟,然后又回到黄灯。游戏可以一直循环下去,直到玩家点击关闭按钮。游戏的图像素材可以自行准备,也可以使用现成的素材。
阅读全文
相关推荐
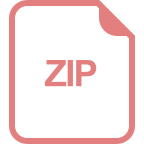
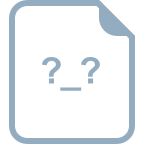
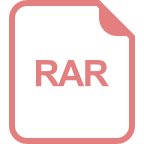
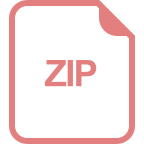
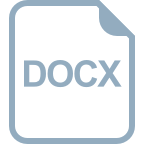
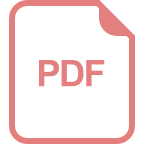
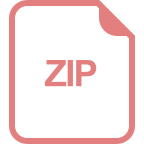
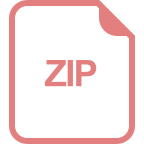
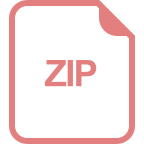
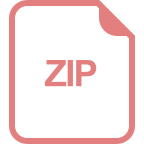
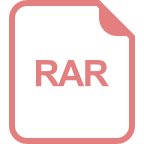