出栈序列的统计 递归c++代码实现
时间: 2023-05-21 16:04:26 浏览: 75
以下是一个递归实现出栈序列的统计代码,可以计算给定的入栈序列和出栈序列是否合法,并且给出合法序列的数量:
```
#include <stdio.h>
// 计算出栈序列的数量
int countPopSequences(int* push, int* pop, int pushLen, int popLen, int pushIndex, int popIndex, int* stack, int stackTop) {
// 如果出栈序列已经结束,说明当前序列合法,直接返回 1
if (popIndex == popLen) return 1;
int count = 0;
// 如果当前栈非空,可以进行出栈操作
if (stackTop >= 0 && stack[stackTop] == pop[popIndex]) {
count += countPopSequences(push, pop, pushLen, popLen, pushIndex, popIndex+1, stack, stackTop-1);
}
// 如果入栈序列还有元素可以入栈,可以进行入栈操作
if (pushIndex < pushLen) {
stack[++stackTop] = push[pushIndex];
count += countPopSequences(push, pop, pushLen, popLen, pushIndex+1, popIndex, stack, stackTop);
--stackTop;
}
return count;
}
int main() {
int push[] = {1, 2, 3, 4, 5};
int pop[] = {5, 4, 3, 2, 1};
int stack[5] = {0};
printf("Pop sequences count: %d\n", countPopSequences(push, pop, 5, 5, 0, 0, stack, -1));
return 0;
}
```
这段代码使用递归实现了出栈序列的统计,它的时间复杂度为 O(2^n),其中 n 为入栈序列长度,因此只适用于小规模问题。如果需要处理大规模问题,需要使用其它更加高效的算法。
此外,我不清楚"lua closure factory 完整代码"和"中文加密"问题的具体信息,无法回答。
相关推荐
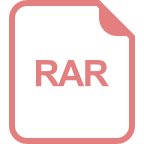
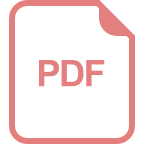














