<% String code = String.valueOf(request.getParameter("YYM")); System.out.print(code); if (code == null || code.isEmpty()) { %> <a href="Admin.jsp">请输入预约码,点击返回签到</a> <% } else { Connection conn = SQLConn.openDB(); final String SELECT_SQL = "SELECT ACode FROM User_ZC JOIN YuYue ON User_ZC.UserId = YuYue.UserId WHERE Login = 1 AND State = 1 AND Arrive IS NULL"; final String UPDATE_SQL = "UPDATE YuYue SET Arrive =1 WHERE ACode = ?"; PreparedStatement pstmtSelect = conn.prepareStatement(SELECT_SQL); ResultSet rs = pstmtSelect.executeQuery(); int count = 0; while (rs.next()) { String Acode = rs.getString("ACode"); if (code==Acode) { try { PreparedStatement pstmtUpdate = conn.prepareStatement(UPDATE_SQL); pstmtUpdate.setString(1, code); count = pstmtUpdate.executeUpdate(); pstmtUpdate.close(); } catch (SQLException e) { e.printStackTrace(); } break; }else{ System.out.print("预约码不匹配"); } } if (count > 0){ %> <a href="Admin.jsp">签到成功</a> <% } else { %> <a href="Admin.jsp">签到失败</a> <% } rs.close(); pstmtSelect.close(); conn.close(); } %>为什么输入正确的YYM,也会提示“预约码不匹配”
时间: 2024-02-14 07:07:40 浏览: 112
在Java中,当比较两个字符串对象时,应该使用equals()方法,而不是"=="运算符。在这段代码中,比较code和Acode的地方使用了"=="运算符,这可能会导致比较结果不正确。正确的方式是使用equals()方法进行比较,即将
```
if (code==Acode) {
```
改为
```
if (code.equals(Acode)) {
```
这样就可以正确地比较code和Acode的内容是否相同了。
相关问题
<% String code = request.getParameter("YYM"); if (code == null || code.isEmpty()) { %> <a href="Admin.jsp">请输入预约码,点击返回签到</a> <% } else { Connection conn = SQLConn.openDB(); final String SELECT_SQL = "SELECT ACode FROM User_ZC JOIN YuYue ON User_ZC.UserId = YuYue.UserId WHERE Login = 1 AND State = 1 AND Arrive IS NULL"; final String UPDATE_SQL = "UPDATE YuYue SET Arrive =1 WHERE ACode = ?"; PreparedStatement pstmtSelect = conn.prepareStatement(SELECT_SQL); ResultSet rs = pstmtSelect.executeQuery(); int count = 0; while (rs.next()) { String Acode = rs.getString("ACode"); if (Acode.equals(code)) { try { PreparedStatement pstmtUpdate = conn.prepareStatement(UPDATE_SQL); pstmtUpdate.setString(1, code); count = pstmtUpdate.executeUpdate(); pstmtUpdate.close(); } catch (SQLException e) { e.printStackTrace(); } break; }else{ System.out.print("预约码不匹配"); } } if (count > 0){ %> <a href="Admin.jsp">签到成功</a> <% } else { %> <a href="Admin.jsp">签到失败</a> <% } rs.close(); pstmtSelect.close(); conn.close(); } %>其中,输入的“YYM”值为003004,并且数据库中有该字段,为什么会跳到“签到失败”页面?
根据代码,如果输入的“YYM”值在数据库中匹配成功,会执行UPDATE_SQL更新操作,如果更新成功,会跳转到“签到成功”页面,否则会跳转到“签到失败”页面。如果您输入的“YYM”值为003004,但是跳转到了“签到失败”页面,可能是因为数据库中的状态不符合要求,例如State不为1或者Arrive不为NULL。建议您检查一下数据库中的状态是否正确,以及程序中的逻辑是否正确。您也可以在程序中增加一些调试语句,例如输出SELECT_SQL查询结果,以便更好地定位问题。
编写程序,实现对数据库StudentInfo中的表student进行插入操作。要求使用预处理语句对象PreparedStatement完成以下数据的插入: insert into student values('001','张三',18,'女') 注:给定数据库登录用户名:sa,密码:123。 Input.jsp: <body> <form action = "addStudent.jsp" method = "post"> 请输入待新增的学生信息<br> 学号:<input type="text" name="sno"/><br> 姓名:<input type="text" name= "sname"/><br> 年龄:<input type="text" name= "sage"/><br> 性别:<input type="text" name= "sex"/><br> <input type = "submit" value= "新增"/> </form> </body> addStudent.jsp: <body> <% String url="jdbc:sqlserver://localhost;databaseName=StudentInfo"; request.setCharacterEncoding("utf-8"); String sno = request.getParameter("sno"); String sname = request.getParameter("sname"); int sage = Integer.valueOf(request.getParameter("sage")); String sex = request.getParameter("sex"); try{ //补全此部分代码 } // 捕获异常 catch(SQLException ex) { System.out.println ("\n*** 发生SQL异常 ***\n"+ex.getMessage()); } catch(ClassNotFoundException ex) { System.out.println(ex); }
补全代码如下:
```java
<%
String url="jdbc:sqlserver://localhost;databaseName=StudentInfo";
request.setCharacterEncoding("utf-8");
String sno = request.getParameter("sno");
String sname = request.getParameter("sname");
int sage = Integer.valueOf(request.getParameter("sage"));
String sex = request.getParameter("sex");
try{
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
Connection conn = DriverManager.getConnection(url,"sa","123");
String sql = "insert into student values(?,?,?,?)";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, sno);
pstmt.setString(2, sname);
pstmt.setInt(3, sage);
pstmt.setString(4, sex);
pstmt.executeUpdate();
out.println("新增成功");
conn.close();
}
// 捕获异常
catch(SQLException ex) {
System.out.println ("\n*** 发生SQL异常 ***\n"+ex.getMessage());
}
catch(ClassNotFoundException ex) {
System.out.println(ex);
}
%>
```
阅读全文
相关推荐
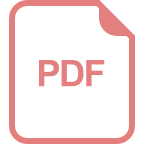
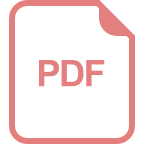
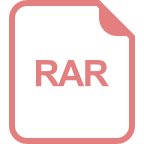


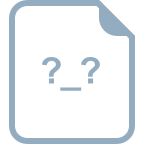
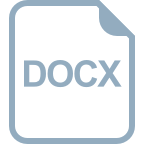
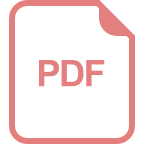
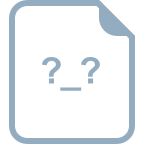
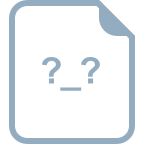
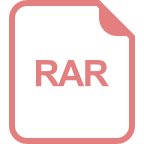
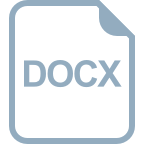
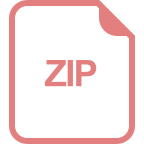

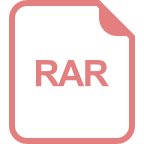
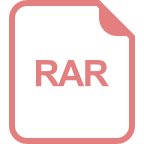