用vue2写一个打地鼠游戏
时间: 2024-03-13 09:09:29 浏览: 72
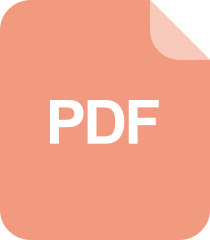
vue实现打地鼠小游戏
下面是一个使用Vue2编写的简单打地鼠游戏的示例:
```html
<!DOCTYPE html>
<html>
<head>
<title>打地鼠游戏</title>
<style>
.hole {
width: 100px;
height: 100px;
background-color: #999;
border: 2px solid #000;
display: flex;
align-items: center;
justify-content: center;
cursor: pointer;
}
.mole {
width: 50px;
height: 50px;
background-color: #333;
border-radius: 50%;
}
</style>
</head>
<body>
<div id="app">
<h1>打地鼠游戏</h1>
<p>得分:{{ score }}</p>
<div>
<div v-for="(hole, index) in holes" :key="index" @click="hitMole(index)">
<div v-if="hole === true" class="hole">
<div class="mole"></div>
</div>
<div v-else class="hole"></div>
</div>
</div>
<button @click="startGame" :disabled="playing">开始游戏</button>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
new Vue({
el: '#app',
data: {
holes: [],
score: 0,
playing: false,
timer: null,
duration: 60
},
methods: {
startGame() {
this.score = 0;
this.playing = true;
this.holes = Array(9).fill(false);
this.timer = setInterval(this.showMole, 1000);
setTimeout(this.endGame, this.duration * 1000);
},
showMole() {
const randomIndex = Math.floor(Math.random() * this.holes.length);
this.holes[randomIndex] = true;
setTimeout(() => {
this.holes[randomIndex] = false;
}, 500);
},
hitMole(index) {
if (this.holes[index]) {
this.score++;
this.holes[index] = false;
}
},
endGame() {
clearInterval(this.timer);
this.playing = false;
}
}
});
</script>
</body>
</html>
```
上述示例中,通过Vue实例的data属性来存储游戏的状态,使用v-for指令来遍历生成地鼠洞,使用v-if指令来控制地鼠的显示和隐藏。点击地鼠洞时,通过@click事件触发hitMole方法来判断是否击中地鼠,更新得分。startGame方法用于开始游戏,showMole方法用于随机显示地鼠,endGame方法用于结束游戏。
请注意,这只是一个简单的示例,游戏的细节和规则可以根据自己的需求进行扩展和修改。
阅读全文
相关推荐
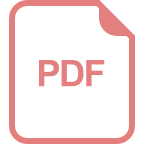
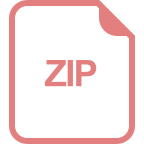
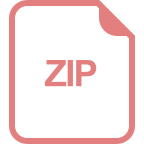
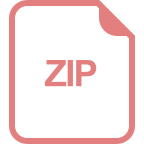
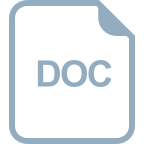
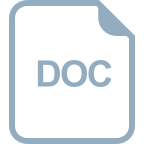
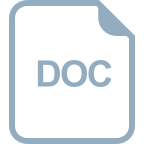
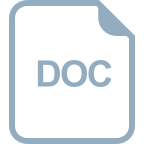
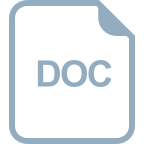
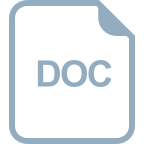
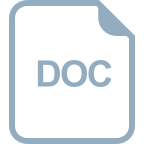
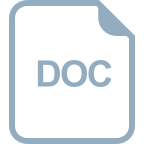
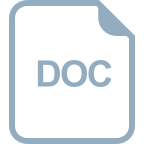
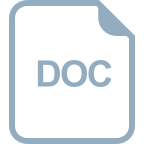

