定义一个 Student 类,其中包含学号、姓名、成绩数据成员。建立若干个 Student 类 对象,将它们保存到文件 Record.dat 中,然后显示文件中的内容。
时间: 2024-02-20 17:56:13 浏览: 82
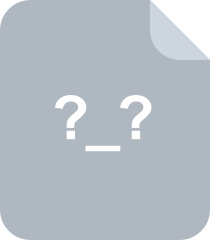
一个 Student类:包括姓名、学号、性别、专业、家庭地址。
好的,这是一个关于文件操作和类的问题。下面是代码实现:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
class Student {
private:
string id; // 学号
string name; // 姓名
int score; // 成绩
public:
Student(string i, string n, int s) : id(i), name(n), score(s) {}
void show() {
cout << "学号:" << id << ",姓名:" << name << ",成绩:" << score << endl;
}
};
int main() {
// 创建学生对象
Student s1("001", "张三", 80);
Student s2("002", "李四", 90);
Student s3("003", "王五", 70);
// 写入文件
ofstream out("Record.dat", ios::out | ios::binary);
if (!out.is_open()) {
cout << "文件打开失败!" << endl;
return 0;
}
out.write((char*)&s1, sizeof(Student));
out.write((char*)&s2, sizeof(Student));
out.write((char*)&s3, sizeof(Student));
out.close();
// 读取文件
ifstream in("Record.dat", ios::in | ios::binary);
if (!in.is_open()) {
cout << "文件打开失败!" << endl;
return 0;
}
Student s;
while (in.read((char*)&s, sizeof(Student))) {
s.show();
}
in.close();
return 0;
}
```
这是一个简单的实现,首先定义了一个 `Student` 类,包含学号、姓名、成绩三个数据成员和一个 `show()` 函数用于显示学生信息。然后在 `main()` 函数中创建了三个学生对象,并将它们依次写入文件 `Record.dat` 中。最后再从文件中读取学生信息,并逐个输出。需要注意的是,这里使用了二进制文件的读写方式,因为文件中保存的是对象的二进制数据,而不是文本数据。
阅读全文
相关推荐
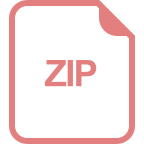
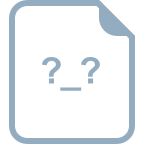
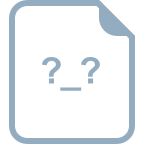
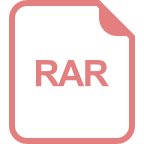
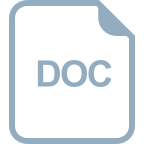
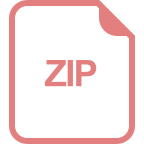
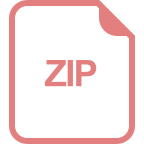
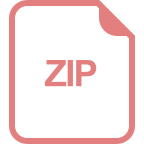
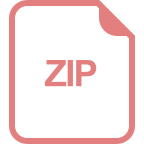
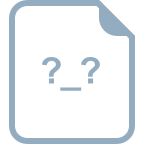
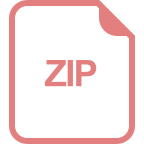