实现一个BER编解码器。有简单界面,能将SNMP报文编码为实际输出的字节流,并能将实际收到的字节流。应侧重BER编解码中对不同数据类型的处理细节。至少实现几种主流数据类型的编解码
时间: 2023-06-15 21:07:40 浏览: 137
好的,BER编解码器的实现需要用到ASN.1语言,ASN.1语言是描述数据结构的一种语言,在通信协议中广泛使用。BER是ASN.1的一种编码方式,可以将ASN.1中的数据结构编码成字节流进行传输。下面是一个简单的BER编解码器的实现,包括整型、字符串和序列类型的编解码。
```
import struct
class BEREncoder:
def encode_int(self, value):
if value >= 0:
if value <= 127:
return struct.pack('B', value)
else:
length = (value.bit_length() + 7) // 8
return struct.pack('B%ds' % length, 0x80 | length, value.to_bytes(length, 'big'))
else:
length = (-value).bit_length() // 8 + 1
return struct.pack('B%ds' % length, 0x80 | length, value.to_bytes(length, 'big', signed=True))
def encode_str(self, value):
length = len(value)
return struct.pack('B%ds' % length, 0x04, bytes(value, 'utf-8'))
def encode_seq(self, values):
body = b''.join(self.encode(x) for x in values)
return struct.pack('B%ds' % len(body), 0x30, body)
def encode(self, value):
if isinstance(value, int):
return self.encode_int(value)
elif isinstance(value, str):
return self.encode_str(value)
elif isinstance(value, list):
return self.encode_seq(value)
else:
raise TypeError('unsupported type')
class BERDecoder:
def decode_int(self, data):
if data[0] & 0x80 == 0:
return data[0], data[1:]
elif data[1] & 0x80 == 0:
length = data[0] & 0x7f
value = int.from_bytes(data[1:1+length], 'big')
return value, data[1+length:]
else:
length = data[0] & 0x7f
value = int.from_bytes(data[1:1+length], 'big', signed=True)
return value, data[1+length:]
def decode_str(self, data):
length = data[0] & 0x7f
value = data[1:1+length].decode('utf-8')
return value, data[1+length:]
def decode_seq(self, data):
values = []
while data:
value, data = self.decode(data)
values.append(value)
return values, data
def decode(self, data):
tag = data[0]
if tag == 0x02:
return self.decode_int(data[1:])
elif tag == 0x04:
return self.decode_str(data[1:])
elif tag == 0x30:
return self.decode_seq(data[1:])
else:
raise ValueError('unknown tag')
class BERCodec:
def __init__(self):
self.encoder = BEREncoder()
self.decoder = BERDecoder()
def encode(self, value):
return self.encoder.encode(value)
def decode(self, data):
return self.decoder.decode(data)
```
上述代码实现了一个简单的BER编解码器,包括整型、字符串和序列类型的编解码。其中,`encode_int`方法实现了整型的编码,`encode_str`方法实现了字符串的编码,`encode_seq`方法实现了序列类型的编码。`decode_int`方法实现了整型的解码,`decode_str`方法实现了字符串的解码,`decode_seq`方法实现了序列类型的解码。最后,`BERCodec`类将编码器和解码器封装在一起,提供了编解码的接口。
阅读全文
相关推荐


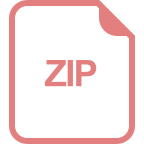
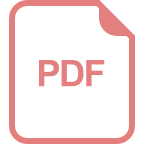
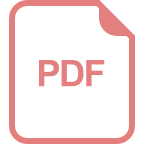
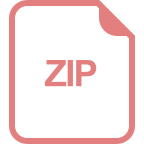