Java编写图形用户界面的图书借阅系统
时间: 2023-09-26 21:09:34 浏览: 49
好的,我会提供一个简单的Java图书借阅系统的代码示例,供您参考。
首先,需要使用Java的GUI库,这里我们选择使用Swing。接下来,我们需要设计数据库的结构,这里我们使用MySQL作为数据库。在MySQL中,我们需要创建三张表:books、users、borrow_records,用于存储书籍信息、用户信息和借阅记录。
books表结构如下:
```
CREATE TABLE books (
id INT(11) NOT NULL AUTO_INCREMENT,
name VARCHAR(50) NOT NULL,
author VARCHAR(50) NOT NULL,
publish_date DATE NOT NULL,
price DOUBLE NOT NULL,
stock INT(11) NOT NULL,
PRIMARY KEY (id)
);
```
users表结构如下:
```
CREATE TABLE users (
id INT(11) NOT NULL AUTO_INCREMENT,
username VARCHAR(50) NOT NULL,
password VARCHAR(50) NOT NULL,
type INT(11) NOT NULL,
PRIMARY KEY (id)
);
```
borrow_records表结构如下:
```
CREATE TABLE borrow_records (
id INT(11) NOT NULL AUTO_INCREMENT,
user_id INT(11) NOT NULL,
book_id INT(11) NOT NULL,
borrow_date DATE NOT NULL,
return_date DATE DEFAULT NULL,
PRIMARY KEY (id),
FOREIGN KEY (user_id) REFERENCES users(id),
FOREIGN KEY (book_id) REFERENCES books(id)
);
```
接下来,我们需要编写Java代码,使用Swing库实现图书借阅系统。
首先,我们需要创建一个登录界面,让用户输入用户名和密码以进行登录。代码如下:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class LoginFrame extends JFrame {
private JLabel userLabel;
private JTextField userField;
private JLabel passwordLabel;
private JPasswordField passwordField;
private JButton loginButton;
public LoginFrame() {
setTitle("图书借阅系统登录");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
userLabel = new JLabel("用户名:");
userField = new JTextField(20);
passwordLabel = new JLabel("密码:");
passwordField = new JPasswordField(20);
loginButton = new JButton("登录");
setLayout(new GridLayout(3, 2));
add(userLabel);
add(userField);
add(passwordLabel);
add(passwordField);
add(new JLabel());
add(loginButton);
loginButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String username = userField.getText();
String password = new String(passwordField.getPassword());
if (validateUser(username, password)) {
dispose();
new MainFrame();
} else {
JOptionPane.showMessageDialog(LoginFrame.this, "用户名或密码错误!", "错误", JOptionPane.ERROR_MESSAGE);
}
}
});
pack();
setLocationRelativeTo(null);
setVisible(true);
}
private boolean validateUser(String username, String password) {
// TODO: 验证用户名和密码
return true;
}
public static void main(String[] args) {
new LoginFrame();
}
}
```
在登录成功后,我们需要创建一个主界面,用于实现图书借阅、归还和查询等功能。代码如下:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class MainFrame extends JFrame {
private JTabbedPane tabbedPane;
public MainFrame() {
setTitle("图书借阅系统");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
tabbedPane = new JTabbedPane();
BooksPanel booksPanel = new BooksPanel();
BorrowPanel borrowPanel = new BorrowPanel();
ReturnPanel returnPanel = new ReturnPanel();
QueryPanel queryPanel = new QueryPanel();
tabbedPane.addTab("图书管理", booksPanel);
tabbedPane.addTab("借阅管理", borrowPanel);
tabbedPane.addTab("归还管理", returnPanel);
tabbedPane.addTab("查询统计", queryPanel);
add(tabbedPane);
pack();
setLocationRelativeTo(null);
setVisible(true);
}
public static void main(String[] args) {
new MainFrame();
}
}
```
然后,我们需要编写BooksPanel、BorrowPanel、ReturnPanel和QueryPanel等面板类,用于实现具体的功能。这里以BooksPanel为例,代码如下:
```
import javax.swing.*;
import javax.swing.table.DefaultTableModel;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.*;
import java.util.Vector;
public class BooksPanel extends JPanel {
private JTable booksTable;
private DefaultTableModel booksTableModel;
private JButton addButton;
private JButton editButton;
private JButton deleteButton;
public BooksPanel() {
setLayout(new BorderLayout());
booksTableModel = new DefaultTableModel();
booksTableModel.addColumn("编号");
booksTableModel.addColumn("书名");
booksTableModel.addColumn("作者");
booksTableModel.addColumn("出版日期");
booksTableModel.addColumn("价格");
booksTableModel.addColumn("库存");
booksTable = new JTable(booksTableModel);
JScrollPane scrollPane = new JScrollPane(booksTable);
add(scrollPane, BorderLayout.CENTER);
JPanel buttonPanel = new JPanel();
addButton = new JButton("添加");
editButton = new JButton("编辑");
deleteButton = new JButton("删除");
buttonPanel.add(addButton);
buttonPanel.add(editButton);
buttonPanel.add(deleteButton);
add(buttonPanel, BorderLayout.SOUTH);
addButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String name = JOptionPane.showInputDialog(BooksPanel.this, "请输入书名:");
String author = JOptionPane.showInputDialog(BooksPanel.this, "请输入作者:");
String publishDate = JOptionPane.showInputDialog(BooksPanel.this, "请输入出版日期(yyyy-MM-dd):");
double price = Double.parseDouble(JOptionPane.showInputDialog(BooksPanel.this, "请输入价格:"));
int stock = Integer.parseInt(JOptionPane.showInputDialog(BooksPanel.this, "请输入库存:"));
Connection connection = null;
PreparedStatement statement = null;
try {
Class.forName("com.mysql.jdbc.Driver");
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/bookdb", "root", "123456");
String sql = "INSERT INTO books (name, author, publish_date, price, stock) VALUES (?, ?, ?, ?, ?)";
statement = connection.prepareStatement(sql);
statement.setString(1, name);
statement.setString(2, author);
statement.setDate(3, Date.valueOf(publishDate));
statement.setDouble(4, price);
statement.setInt(5, stock);
statement.executeUpdate();
loadBooks();
} catch (SQLException ex) {
ex.printStackTrace();
JOptionPane.showMessageDialog(BooksPanel.this, "添加失败!", "错误", JOptionPane.ERROR_MESSAGE);
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
} finally {
try {
if (statement != null) statement.close();
if (connection != null) connection.close();
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
});
editButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int rowIndex = booksTable.getSelectedRow();
if (rowIndex == -1) {
JOptionPane.showMessageDialog(BooksPanel.this, "请选择要编辑的书籍!", "提示", JOptionPane.WARNING_MESSAGE);
return;
}
int id = (int) booksTableModel.getValueAt(rowIndex, 0);
String name = JOptionPane.showInputDialog(BooksPanel.this, "请输入书名:", booksTableModel.getValueAt(rowIndex, 1));
String author = JOptionPane.showInputDialog(BooksPanel.this, "请输入作者:", booksTableModel.getValueAt(rowIndex, 2));
String publishDate = JOptionPane.showInputDialog(BooksPanel.this, "请输入出版日期(yyyy-MM-dd):", booksTableModel.getValueAt(rowIndex, 3));
double price = Double.parseDouble(JOptionPane.showInputDialog(BooksPanel.this, "请输入价格:", booksTableModel.getValueAt(rowIndex, 4)));
int stock = Integer.parseInt(JOptionPane.showInputDialog(BooksPanel.this, "请输入库存:", booksTableModel.getValueAt(rowIndex, 5)));
Connection connection = null;
PreparedStatement statement = null;
try {
Class.forName("com.mysql.jdbc.Driver");
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/bookdb", "root", "123456");
String sql = "UPDATE books SET name=?, author=?, publish_date=?, price=?, stock=? WHERE id=?";
statement = connection.prepareStatement(sql);
statement.setString(1, name);
statement.setString(2, author);
statement.setDate(3, Date.valueOf(publishDate));
statement.setDouble(4, price);
statement.setInt(5, stock);
statement.setInt(6, id);
statement.executeUpdate();
loadBooks();
} catch (SQLException ex) {
ex.printStackTrace();
JOptionPane.showMessageDialog(BooksPanel.this, "编辑失败!", "错误", JOptionPane.ERROR_MESSAGE);
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
} finally {
try {
if (statement != null) statement.close();
if (connection != null) connection.close();
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
});
deleteButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int rowIndex = booksTable.getSelectedRow();
if (rowIndex == -1) {
JOptionPane.showMessageDialog(BooksPanel.this, "请选择要删除的书籍!", "提示", JOptionPane.WARNING_MESSAGE);
return;
}
int id = (int) booksTableModel.getValueAt(rowIndex, 0);
Connection connection = null;
PreparedStatement statement = null;
try {
Class.forName("com.mysql.jdbc.Driver");
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/bookdb", "root", "123456");
String sql = "DELETE FROM books WHERE id=?";
statement = connection.prepareStatement(sql);
statement.setInt(1, id);
statement.executeUpdate();
loadBooks();
} catch (SQLException ex) {
ex.printStackTrace();
JOptionPane.showMessageDialog(BooksPanel.this, "删除失败!", "错误", JOptionPane.ERROR_MESSAGE);
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
} finally {
try {
if (statement != null) statement.close();
if (connection != null) connection.close();
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
});
loadBooks();
}
private void loadBooks() {
Connection connection = null;
Statement statement = null;
try {
Class.forName("com.mysql.jdbc.Driver");
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/bookdb", "root", "123456");
String sql = "SELECT * FROM books";
statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery(sql);
booksTableModel.setRowCount(0);
while (resultSet.next()) {
Vector<Object> rowData = new Vector<>();
rowData.add(resultSet.getInt("id"));
rowData.add(resultSet.getString("name"));
rowData.add(resultSet.getString("author"));
rowData.add(resultSet.getDate("publish_date"));
rowData.add(resultSet.getDouble("price"));
rowData.add(resultSet.getInt("stock"));
booksTableModel.addRow(rowData);
}
} catch (SQLException ex) {
ex.printStackTrace();
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
} finally {
try {
if (statement != null) statement.close();
if (connection != null) connection.close();
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
}
```
这里只展示了BooksPanel的代码,其他面板类的代码类似。需要注意的是,在代码中需要使用JDBC连接数据库进行数据的增删改查操作,同时需要注意异常处理和数据类型转换等问题。
希望这个例子能够帮助您了解Java图书借阅系统的开发过程和技术点。
相关推荐
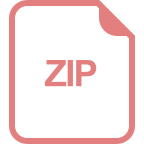
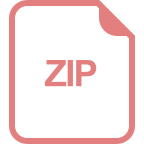
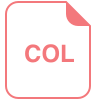
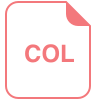












