Jupyter爬取找属性值
时间: 2024-05-25 08:04:57 浏览: 19
您可以使用Python的requests库和BeautifulSoup库来爬取网页并找到属性值。以下是一个示例代码:
首先,导入所需的库:
```python
import requests
from bs4 import BeautifulSoup
```
然后,使用requests库获取网页的HTML代码:
```python
url = 'https://www.example.com'
response = requests.get(url)
html = response.content
```
接下来,使用BeautifulSoup库解析HTML代码,找到所需的属性值:
```python
soup = BeautifulSoup(html, 'html.parser')
tag = soup.find('div', {'class': 'my-class'}) # 找到class为'my-class'的div标签
attribute_value = tag['my-attribute'] # 获取这个标签的'my-attribute'属性的值
```
请注意,以上代码中的`'https://www.example.com'`和`'my-class'`是示例URL和属性值,您需要将它们替换为您要爬取的实际网页和属性值。
希望这个示例能够帮助您完成所需的任务。
相关问题
jupyter爬取虎扑球员
Jupyter Notebook 是一个强大的交互式计算环境,常用于数据科学和机器学习项目中。要使用 Jupyter 爬取虎扑(Hupu)的数据,特别是关于球员的信息,你需要遵循这些步骤:
1. 安装必要的库:首先,确保你已经安装了 Python 的爬虫库,如 `requests`, `BeautifulSoup` 或 `pandas` 用于处理网页抓取和数据解析。
```python
!pip install requests beautifulsoup4 pandas
```
2. 导入库并导入相关模块:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
```
3. 设定目标 URL:找到包含球员信息的虎扑页面的URL。通常,虎扑的数据可能没有直接的 API 提供,所以可能需要手动查找并分析HTML结构。
4. 发送请求并解析响应:
```python
url = "https://www.hupu.com/player/{}" # 用实际球员ID替换这里的 {}
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
```
5. 提取数据:使用 BeautifulSoup 解析 HTML 并提取需要的球员信息,这可能包括名字、位置、统计数据等。具体元素定位可能依赖于网站的具体结构。
```python
player_info = soup.find_all('div', class_='some_class') # 'some_class' 需要根据实际HTML结构替换
data = []
for info in player_info:
name = info.find('span', class_='name').text
position = info.find('span', class_='position').text
# ...其他数据提取
data.append([name, position, ...]) # 根据实际情况填充数据字段
```
6. 将数据保存为 DataFrame 或 CSV 文件:
```python
df = pd.DataFrame(data, columns=['Name', 'Position', ...])
df.to_csv('player_data.csv', index=False)
```
7. 注意事项:
- 网页结构可能会经常变化,导致解析代码失效,所以需要定期检查或使用动态解析(如 Selenium 或 Scrapy)。
- 虎扑或其他网站可能有反爬虫策略,如验证码、频率限制等,爬取时需遵守网站的使用协议,尊重机器人协议(robots.txt)。
用jupyter爬取微博关键词
当使用Jupyter进行微博关键词爬取时,你可以按照以下步骤进行操作:
1. 安装必要的库:首先,你需要安装Python的requests、beautifulsoup4和pandas库。你可以使用以下命令来安装它们:
```
pip install requests beautifulsoup4 pandas
```
2. 导入所需的库:在Jupyter Notebook中,你需要导入这些库以便使用它们。使用以下代码导入它们:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
```
3. 发送请求并解析页面:使用requests库发送HTTP请求,并使用BeautifulSoup库解析返回的HTML页面。以下是一个示例代码:
```python
# 发送请求
url = 'https://s.weibo.com/weibo?q=关键词'
response = requests.get(url)
# 解析页面
soup = BeautifulSoup(response.text, 'html.parser')
```
请将"关键词"替换为你要搜索的实际关键词。
4. 提取所需信息:根据页面的HTML结构,使用BeautifulSoup库提取你需要的信息。例如,如果你想提取微博的文本内容和发布时间,可以使用以下代码:
```python
# 提取微博内容和发布时间
weibo_list = soup.find_all('div', class_='content')
for weibo in weibo_list:
text = weibo.find('p').text.strip()
time = weibo.find('p', class_='from').find('a').text.strip()
print(text, time)
```
5. 存储数据:如果你想将提取的数据保存到文件中,可以使用pandas库将数据转换为DataFrame,并将其保存为CSV文件。以下是一个示例代码:
```python
# 创建DataFrame并保存为CSV文件
data = {'Text': [], 'Time': []}
for weibo in weibo_list:
text = weibo.find('p').text.strip()
time = weibo.find('p', class_='from').find('a').text.strip()
data['Text'].append(text)
data['Time'].append(time)
df = pd.DataFrame(data)
df.to_csv('weibo_data.csv', index=False)
```
请确保你已经创建了一个名为"weibo_data.csv"的文件。
相关推荐
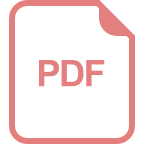
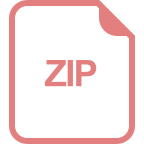












