JS9.编写inherit()函数,它返回了一个继承自原型对象p的属性的新对象,这里使用ECMAScript 5中的Object.create()函数(如果存在的话),如果不存在Object.create(),则退化使用其他方法
时间: 2024-05-09 07:14:30 浏览: 78
可以这样实现:
```javascript
function inherit(p) {
if (p == null) throw TypeError(); // p不能为null或undefined
// 如果Object.create()存在,则使用它
if (Object.create) {
return Object.create(p);
}
// 否则,退化使用其他方法
var t = typeof p;
if (t !== "object" && t !== "function") throw TypeError();
function f() {}
f.prototype = p;
return new f();
}
```
使用示例:
```javascript
var o = { x: 1 };
var p = inherit(o);
p.y = 2;
console.log(p.x); // 1
console.log(p.y); // 2
console.log(o.hasOwnProperty("y")); // false,没有继承自p的属性
```
相关问题
JS 编写inherit()函数,它返回了一个继承自原型对象p的属性的新对象,这里使用ECMAScript 5中的Object.create()函数(如果存在的话),如果不存在Object.create(),则退化使用其他方法
```javascript
function inherit(p) {
if (p == null) throw TypeError(); // p不能为空
// 如果Object.create()存在,则使用它
if (Object.create) return Object.create(p);
// 否则,使用其他方法
var t = typeof p;
if (t !== "object" && t !== "function") throw TypeError();
function f() {}
f.prototype = p;
return new f();
}
```
该函数先检查传入的参数p是否为空,如果为空则抛出一个类型错误的异常。然后判断当前环境下是否支持Object.create()函数,如果支持则直接使用它来创建一个新对象并继承原型对象p的属性。如果不支持,则先检查p的类型,如果不是对象或函数则抛出一个类型错误的异常,否则创建一个空函数f,并将f的原型对象设置为p,最后使用new操作符来创建一个继承自p的新对象。
function ContinuousForceDirectedLayout() { go.ForceDirectedLayout.call(this); this._isObserving = false; this.defaultDirection = go.LayoutDirection.Right; } // 继承go.ForceDirectedLayout的原型 go.Diagram.inherit(ContinuousForceDirectedLayout, go.ForceDirectedLayout); // 将类的构造函数设置为ContinuousForceDirectedLayout ContinuousForceDirectedLayout.prototype.constructor = ContinuousForceDirectedLayout;报错如下Uncaught TypeError: Cannot read properties of undefined (reading 'Right')
在使用 `ContinuousForceDirectedLayout` 类之前,需要确保 GoJS 库已经正确加载并被定义。同时,需要确保 `go.LayoutDirection` 已经被定义。根据你提供的错误信息,可能是 `go.LayoutDirection` 没有被正确定义,导致无法读取 `Right` 属性。
你可以在代码中添加以下语句,以检查 `go.LayoutDirection` 是否已经被定义:
```
console.log(go.LayoutDirection);
```
如果输出结果为 `undefined`,说明 `go.LayoutDirection` 还未被定义。你需要在 `<script>` 标签中添加以下代码,以定义 `go.LayoutDirection`:
```
var go = go || {};
go.LayoutDirection = { Down: 0, Right: 1, Left: 2, Up: 3 };
```
这样就可以确保 `go.LayoutDirection` 被正确定义,并且 `defaultDirection` 属性可以被正确设置。
阅读全文
相关推荐
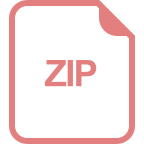
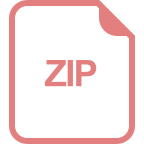
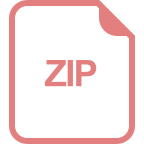
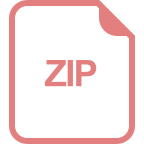
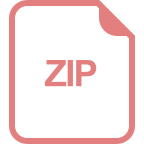
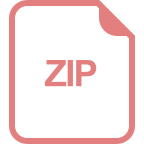
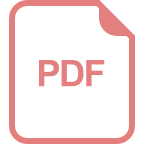
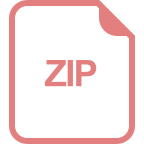
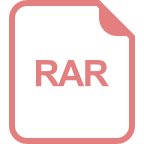
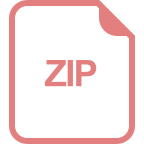
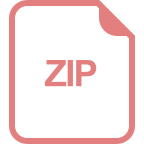
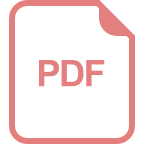
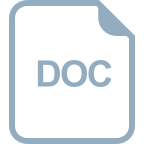
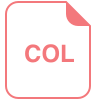
