给定N个学生的基本信息,包括学号(由5个数字组成的字符串)、姓名(长度小于10的不包含空白字符的非空字符串)和3门课程的成绩([0,100]区间内的整数),要求输出总分最高学生的姓名、学号和总分
时间: 2023-12-08 13:19:52 浏览: 184
好的,根据您的要求,以下是解决方案:
您可以使用结构体来存储学生信息,然后使用一个数组来存储所有学生的信息。同时,您可以使用一个变量来记录当前总分最高的学生的学号、姓名和总分。
以下是 C++ 代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
struct Student {
string id;
string name;
int score[3];
int total_score;
};
int main() {
int n;
cin >> n;
Student students[n];
int max_score = -1;
string max_id, max_name;
for (int i = 0; i < n; i++) {
cin >> students[i].id >> students[i].name;
students[i].total_score = 0;
for (int j = 0; j < 3; j++) {
cin >> students[i].score[j];
students[i].total_score += students[i].score[j];
}
if (students[i].total_score > max_score) {
max_score = students[i].total_score;
max_id = students[i].id;
max_name = students[i].name;
}
}
cout << max_name << " " << max_id << " " << max_score << endl;
return 0;
}
```
以上代码中,我们首先输入学生数量 n,然后定义一个结构体 Student 来存储学生信息,包括学号、姓名、三门课程的成绩和总分。然后我们使用一个数组来存储所有学生的信息,并用一个变量 max_score 来记录当前总分最高的学生的分数。在输入每个学生的信息时,我们将其三门课程的成绩相加,并更新该学生的总分。如果该学生的总分超过了目前最高分数,我们就更新最高分数和对应的学生信息。
最后,我们输出总分最高的学生的姓名、学号和总分。
相关推荐
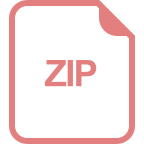
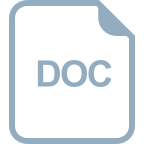
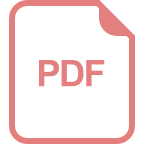









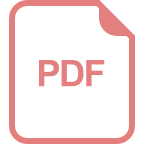
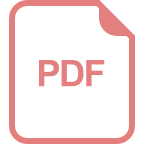
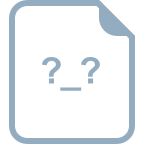
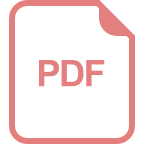
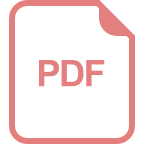
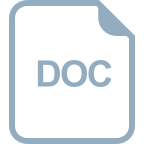
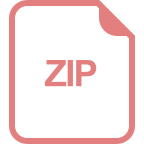