kafka如何保证消息的顺序性 代码
时间: 2023-07-05 11:03:30 浏览: 63
Kafka通过分区(partition)来实现消息的顺序性,每个分区内的消息都是有序的,而不同分区之间的消息是无序的。
当生产者发送消息时,可以指定消息要发送到哪个分区中,如果没有指定,则根据Kafka的分区策略来自动分配。同一个分区内的消息按照发送的顺序依次写入,因此可以保证消息的顺序性。
消费者消费消息时,也是按照分区来消费的,同一个分区内的消息是有序的。消费者可以通过设置参数来保证消费过程中的顺序性,例如设置为只允许一个消费者消费同一个分区中的消息,或者使用同步提交偏移量的方式来保证消费的顺序性。
下面是一个简单的Java代码示例,演示了如何使用Kafka生产和消费有序的消息:
```java
import org.apache.kafka.clients.producer.*;
import org.apache.kafka.clients.consumer.*;
import org.apache.kafka.common.serialization.StringDeserializer;
import org.apache.kafka.common.serialization.StringSerializer;
import java.util.Properties;
public class KafkaOrderDemo {
private static final String TOPIC = "test-topic";
private static final String BOOTSTRAP_SERVERS = "localhost:9092";
public static void main(String[] args) {
// 生产者发送消息
Properties producerProps = new Properties();
producerProps.put(ProducerConfig.BOOTSTRAP_SERVERS_CONFIG, BOOTSTRAP_SERVERS);
producerProps.put(ProducerConfig.KEY_SERIALIZER_CLASS_CONFIG, StringSerializer.class.getName());
producerProps.put(ProducerConfig.VALUE_SERIALIZER_CLASS_CONFIG, StringSerializer.class.getName());
Producer<String, String> producer = new KafkaProducer<>(producerProps);
for (int i = 0; i < 10; i++) {
ProducerRecord<String, String> record = new ProducerRecord<>(TOPIC, "key", "message " + i);
producer.send(record);
}
producer.close();
// 消费者消费消息
Properties consumerProps = new Properties();
consumerProps.put(ConsumerConfig.BOOTSTRAP_SERVERS_CONFIG, BOOTSTRAP_SERVERS);
consumerProps.put(ConsumerConfig.GROUP_ID_CONFIG, "test-group");
consumerProps.put(ConsumerConfig.KEY_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class.getName());
consumerProps.put(ConsumerConfig.VALUE_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class.getName());
consumerProps.put(ConsumerConfig.AUTO_OFFSET_RESET_CONFIG, "earliest");
consumerProps.put(ConsumerConfig.ENABLE_AUTO_COMMIT_CONFIG, false);
consumerProps.put(ConsumerConfig.MAX_POLL_RECORDS_CONFIG, 1);
Consumer<String, String> consumer = new KafkaConsumer<>(consumerProps);
consumer.subscribe(Collections.singleton(TOPIC));
while (true) {
ConsumerRecords<String, String> records = consumer.poll(1000);
for (ConsumerRecord<String, String> record : records) {
System.out.println("Received message: " + record.value());
}
consumer.commitSync();
}
}
}
```
相关推荐
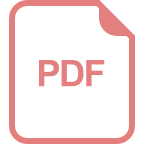
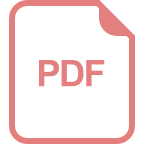












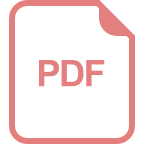