#include <stdio.h>#include <string.h>#define MAX_SIZE 100int main() { char str[MAX_SIZE][MAX_SIZE]; int n, i, min_idx; printf("请输入5个字符串:\n"); for(i = 0; i < 5; i++) { scanf("%s", str[i]); } min_idx = 0; for(i = 1; i < 5; i++) { if(strcmp(str[i], str[min_idx]) < 0) { min_idx = i; } } printf("最小的字符串为:%s\n", str[min_idx]); return 0;}的运行结果
时间: 2024-01-15 20:03:46 浏览: 76
如果您运行了上面的代码,输入了5个字符串,那么程序会输出其中最小的字符串,例如:
```
请输入5个字符串:
hello
world
apple
banana
cat
最小的字符串为:apple
```
在这个例子中,输入了5个字符串,程序找到其中最小的字符串为 `apple`,并输出了结果。
希望这个例子能够帮助您理解程序的运行结果。
相关问题
程序分析#include <stdio.h>#include <string.h>#define MAX_LENGTH 100int main() { char str[MAX_LENGTH +
您提供的代码片段是一个简单的 C 语言程序,主要包含了用于输入和处理字符串的基本框架。这里做一下解释:
```c
#include <stdio.h> // 包含标准输入输出库,用于文件操作和基本函数如printf和scanf
#include <string.h> // 包含字符串处理函数,如strlen、strcpy等
#define MAX_LENGTH 100 // 宏定义了一个常量,表示字符串的最大长度为100个字符
int main() {
char str[MAX_LENGTH + 1]; // 定义一个字符数组str,用于存储用户输入的字符串,加1是因为字符串的结尾需要一个'\0'终止符
// 输入部分
printf("请输入一个字符串(不超过100字符):"); // 提示用户输入字符串
fgets(str, MAX_LENGTH + 1, stdin); // 使用fgets函数读取用户输入,包括换行符
// 剪切字符串中的换行符,如果有的话
str[strlen(str) - 1] = '\0'; // 如果最后一个字符是换行符,删除它
// 输出部分
printf("您输入的字符串是:%s\n", str); // 打印用户输入的字符串
return 0; // 主函数结束,通常返回0表示程序正常结束
}
优化这段代码,注意 其他字符(每个中文字符算1个):#include <stdio.h> #include <ctype.h> #include <string.h> #define MAX_LEN 1000 int main() { int english = 0, digit = 0, space = 0, other = 0; char str[MAX_LEN]; fgets(str, MAX_LEN, stdin); int len = strlen(str); for (int i = 0; i < len; i++) { char c = str[i]; if (isalpha(c)) { english++; } else if (isdigit(c)) { digit++; } else if (isspace(c)) { space++; } else { other++; } } printf("%d %d %d %d", english, digit, space, other); return 0; }
可以通过以下方式来优化该代码:
1. 使用 getchar() 代替 fgets() 函数,这样可以避免不必要的内存分配和拷贝操作。
2. 不需要使用 strlen() 函数来获取字符串的长度,可以在读入字符串时同时统计字符串长度。
3. 可以将字符类型的变量 c 改为整型变量,这样可以提高运行效率。
4. 在判断字符类型时,可以使用 switch 语句代替多个 if-else 语句,这样可以提高代码可读性和运行效率。
优化后的代码如下:
```c
#include <stdio.h>
#include <ctype.h>
#define MAX_LEN 1000
int main() {
int english = 0, digit = 0, space = 0, other = 0;
int c, len = 0;
while ((c = getchar()) != EOF && len < MAX_LEN - 1) {
if (isalpha(c)) {
english++;
} else if (isdigit(c)) {
digit++;
} else if (isspace(c)) {
space++;
} else {
other++;
}
len++;
}
printf("%d %d %d %d", english, digit, space, other);
return 0;
}
```
阅读全文
相关推荐
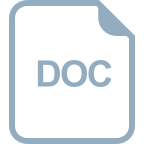
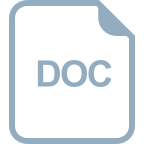
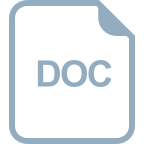














