2、创建jdbc项目,实现数据库连接,并完成如下功能: 1)输入一个部门]的信息,完成部门的添加; 2)根据部门编号针对部门门名称进行修改的功能:输入部门编号和修改 名称的修改! 3)输入一个员工的信息,完成员工的添加。 4)完成如下查询: 4.1)查询所有员工信息; 4.2)输入姓名,查询员工信息; 3、创建jdbc项目,使用逻辑三层结构,把第2题的功能重新实现一遍;
时间: 2023-12-03 20:43:13 浏览: 100
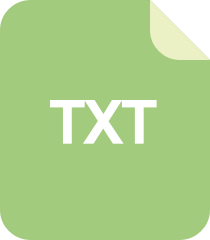
jdbc连接数据库的步骤给大家分享了
很好,这是一个比较完整的jdbc项目,需要分步骤来实现。
1. 创建jdbc项目并连接数据库
首先需要创建一个Java项目,并添加JDBC驱动jar包,然后在代码中连接数据库。
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnection {
private static final String URL = "jdbc:mysql://localhost:3306/demo";
private static final String USER = "root";
private static final String PASSWORD = "root";
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(URL, USER, PASSWORD);
}
}
```
2. 实现部门的添加和修改功能
定义一个`DepartmentDao`接口,里面包含添加和修改部门的方法。
```java
public interface DepartmentDao {
void addDepartment(Department department) throws SQLException;
void updateDepartment(int id, String newName) throws SQLException;
}
```
然后实现接口中的方法。
```java
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class DepartmentDaoImpl implements DepartmentDao {
private Connection connection;
public DepartmentDaoImpl(Connection connection) {
this.connection = connection;
}
@Override
public void addDepartment(Department department) throws SQLException {
String sql = "INSERT INTO department (name) VALUES (?)";
PreparedStatement statement = connection.prepareStatement(sql);
statement.setString(1, department.getName());
statement.executeUpdate();
statement.close();
}
@Override
public void updateDepartment(int id, String newName) throws SQLException {
String sql = "UPDATE department SET name=? WHERE id=?";
PreparedStatement statement = connection.prepareStatement(sql);
statement.setString(1, newName);
statement.setInt(2, id);
statement.executeUpdate();
statement.close();
}
}
```
3. 实现员工的添加和查询功能
定义一个`EmployeeDao`接口,里面包含添加和查询员工的方法。
```java
import java.sql.SQLException;
import java.util.List;
public interface EmployeeDao {
void addEmployee(Employee employee) throws SQLException;
List<Employee> getAllEmployees() throws SQLException;
List<Employee> getEmployeesByName(String name) throws SQLException;
}
```
然后实现接口中的方法。
```java
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
public class EmployeeDaoImpl implements EmployeeDao {
private Connection connection;
public EmployeeDaoImpl(Connection connection) {
this.connection = connection;
}
@Override
public void addEmployee(Employee employee) throws SQLException {
String sql = "INSERT INTO employee (name, age, gender, department_id) VALUES (?, ?, ?, ?)";
PreparedStatement statement = connection.prepareStatement(sql);
statement.setString(1, employee.getName());
statement.setInt(2, employee.getAge());
statement.setString(3, employee.getGender());
statement.setInt(4, employee.getDepartmentId());
statement.executeUpdate();
statement.close();
}
@Override
public List<Employee> getAllEmployees() throws SQLException {
String sql = "SELECT * FROM employee";
PreparedStatement statement = connection.prepareStatement(sql);
ResultSet resultSet = statement.executeQuery();
List<Employee> employees = new ArrayList<>();
while (resultSet.next()) {
Employee employee = new Employee();
employee.setId(resultSet.getInt("id"));
employee.setName(resultSet.getString("name"));
employee.setAge(resultSet.getInt("age"));
employee.setGender(resultSet.getString("gender"));
employee.setDepartmentId(resultSet.getInt("department_id"));
employees.add(employee);
}
resultSet.close();
statement.close();
return employees;
}
@Override
public List<Employee> getEmployeesByName(String name) throws SQLException {
String sql = "SELECT * FROM employee WHERE name=?";
PreparedStatement statement = connection.prepareStatement(sql);
statement.setString(1, name);
ResultSet resultSet = statement.executeQuery();
List<Employee> employees = new ArrayList<>();
while (resultSet.next()) {
Employee employee = new Employee();
employee.setId(resultSet.getInt("id"));
employee.setName(resultSet.getString("name"));
employee.setAge(resultSet.getInt("age"));
employee.setGender(resultSet.getString("gender"));
employee.setDepartmentId(resultSet.getInt("department_id"));
employees.add(employee);
}
resultSet.close();
statement.close();
return employees;
}
}
```
4. 整合以上功能并实现三层结构
现在可以将以上功能整合起来,创建一个`Service`层,提供给外部调用。
```java
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
public class EmployeeService {
private DepartmentDao departmentDao;
private EmployeeDao employeeDao;
public EmployeeService() {
try {
Connection connection = DatabaseConnection.getConnection();
departmentDao = new DepartmentDaoImpl(connection);
employeeDao = new EmployeeDaoImpl(connection);
} catch (SQLException e) {
e.printStackTrace();
}
}
public void addDepartment(Department department) {
try {
departmentDao.addDepartment(department);
} catch (SQLException e) {
e.printStackTrace();
}
}
public void updateDepartment(int id, String newName) {
try {
departmentDao.updateDepartment(id, newName);
} catch (SQLException e) {
e.printStackTrace();
}
}
public void addEmployee(Employee employee) {
try {
employeeDao.addEmployee(employee);
} catch (SQLException e) {
e.printStackTrace();
}
}
public List<Employee> getAllEmployees() {
try {
return employeeDao.getAllEmployees();
} catch (SQLException e) {
e.printStackTrace();
return null;
}
}
public List<Employee> getEmployeesByName(String name) {
try {
return employeeDao.getEmployeesByName(name);
} catch (SQLException e) {
e.printStackTrace();
return null;
}
}
}
```
这里的`Service`层对外提供了四个方法,分别是添加部门、修改部门、添加员工、查询所有员工和根据姓名查询员工。
至此,整个jdbc项目就完成了。
阅读全文
相关推荐
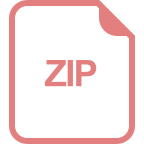
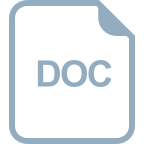

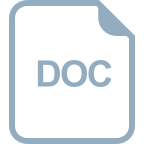
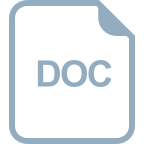
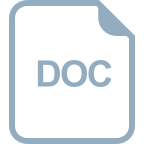
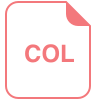
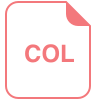
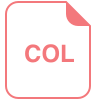
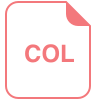
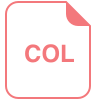
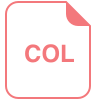
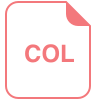
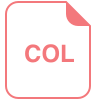


