ajax get请求传递json参数
时间: 2023-04-23 20:07:16 浏览: 121
Ajax GET请求可以通过在URL后面添加参数来传递JSON数据。您可以使用jQuery的$.ajax()方法来实现这个功能,代码如下:
```
$.ajax({
type: "GET",
url: "your_url",
data: JSON.stringify({json_data: "data"}),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(response) {
// Do something with the response
}
});
```
其中,`contentType`设置为`"application/json; charset=utf-8"`,表示请求的数据格式为JSON;`data`字段包含要发送的JSON数据。
相关问题
ajax中post请求传递json参数是怎样的过程
在Ajax中使用POST请求传递JSON参数的过程通常如下:
1. 创建一个 XMLHttpRequest 对象。
2. 使用 XMLHttpRequest 对象的 open() 方法指定请求的方法、URL和是否异步请求。
3. 设置请求头信息 Content-Type 为 application/json。
4. 将JSON对象转换为字符串。
5. 使用 XMLHttpRequest 对象的 send() 方法将 JSON 字符串发送到服务器。
6. 在服务器端收到请求后,将请求体中的 JSON 字符串解析为 JSON 对象进行处理。
7. 将处理结果返回给客户端。
8. 在客户端使用回调函数处理服务器返回的结果。
以下是一个使用jQuery的POST请求传递JSON参数的示例代码:
```
$.ajax({
type: 'POST',
url: 'http://example.com/myservice',
data: JSON.stringify({name:'John', age:30}),
contentType: 'application/json',
success: function(data) {
console.log('success:', data);
},
error: function(xhr, status, error) {
console.log('error:', error);
}
});
```
在上述代码中,`JSON.stringify({name:'John', age:30})`将JSON对象`{name:'John', age:30}`转换为字符串,并通过`data`字段传递给服务器。`contentType: 'application/json'`指定了请求头信息的`Content-Type`为`application/json`。在`success`回调函数中,处理服务器返回的结果。
ajax get 传递json
可以使用 jQuery 的 ajax 方法来传递 JSON 数据。以下是一个示例:
```javascript
$.ajax({
type: "GET",
url: "your_url",
data: { your_data: JSON.stringify(your_json_object) },
success: function(response) {
// 处理响应
},
error: function(jqXHR, textStatus, errorThrown) {
// 处理错误
}
});
```
在上面的示例中,我们使用 `JSON.stringify()` 方法将 JSON 对象转换为字符串,并将其作为 GET 请求的参数传递。在服务器端,您可以使用相应的语言(如 PHP、Python 等)来解析传递的 JSON 数据。
阅读全文
相关推荐
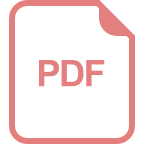
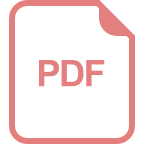
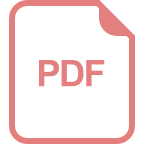













