来个js可运行的贪吃蛇
时间: 2023-08-11 16:05:46 浏览: 62
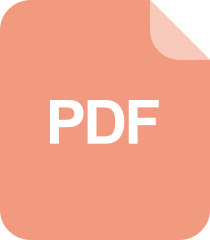
JS实现贪吃蛇游戏
好的,以下是一个简单的基于 Canvas 的 JavaScript 贪吃蛇游戏。你可以在浏览器中运行它。
HTML 代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>贪吃蛇游戏</title>
<style>
canvas {
border: 2px solid black;
}
</style>
</head>
<body>
<canvas id="canvas" width="400" height="400"></canvas>
<script src="snake.js"></script>
</body>
</html>
```
JavaScript 代码(保存为 snake.js 文件):
```javascript
const canvas = document.getElementById("canvas");
const ctx = canvas.getContext("2d");
const TILE_SIZE = 20;
const NUM_ROWS = canvas.height / TILE_SIZE;
const NUM_COLS = canvas.width / TILE_SIZE;
let snake = [{x: 0, y: 0}];
let food = {x: 0, y: 0};
let direction = "right";
let score = 0;
function draw() {
// 清空画布
ctx.fillStyle = "white";
ctx.fillRect(0, 0, canvas.width, canvas.height);
// 画蛇
ctx.fillStyle = "green";
snake.forEach(segment => {
ctx.fillRect(segment.x * TILE_SIZE, segment.y * TILE_SIZE, TILE_SIZE, TILE_SIZE);
});
// 画食物
ctx.fillStyle = "red";
ctx.fillRect(food.x * TILE_SIZE, food.y * TILE_SIZE, TILE_SIZE, TILE_SIZE);
// 显示得分
ctx.fillStyle = "black";
ctx.font = "16px Arial";
ctx.fillText(`Score: ${score}`, 10, 20);
}
function move() {
// 计算新的头部坐标
let newHead = {x: snake[0].x, y: snake[0].y};
switch (direction) {
case "up":
newHead.y--;
break;
case "down":
newHead.y++;
break;
case "left":
newHead.x--;
break;
case "right":
newHead.x++;
break;
}
// 判断是否吃到食物
if (newHead.x === food.x && newHead.y === food.y) {
snake.unshift(newHead);
placeFood();
score++;
} else {
// 移动蛇
snake.pop();
snake.unshift(newHead);
}
// 判断是否撞墙或自咬
if (newHead.x < 0 || newHead.x >= NUM_COLS || newHead.y < 0 || newHead.y >= NUM_ROWS) {
reset();
} else if (snake.slice(1).some(segment => segment.x === newHead.x && segment.y === newHead.y)) {
reset();
}
}
function placeFood() {
food.x = Math.floor(Math.random() * NUM_COLS);
food.y = Math.floor(Math.random() * NUM_ROWS);
}
function reset() {
snake = [{x: 0, y: 0}];
direction = "right";
score = 0;
placeFood();
}
document.addEventListener("keydown", event => {
switch (event.key) {
case "ArrowUp":
if (direction !== "down") {
direction = "up";
}
break;
case "ArrowDown":
if (direction !== "up") {
direction = "down";
}
break;
case "ArrowLeft":
if (direction !== "right") {
direction = "left";
}
break;
case "ArrowRight":
if (direction !== "left") {
direction = "right";
}
break;
}
});
placeFood();
setInterval(() => {
move();
draw();
}, 100);
```
这是一个非常基础的实现,你可以根据自己的需要进行扩展和改进。
阅读全文
相关推荐
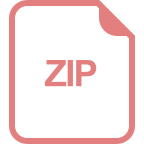
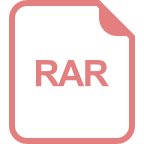
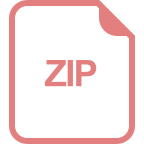
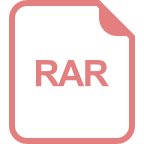
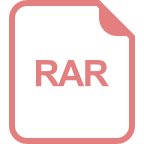
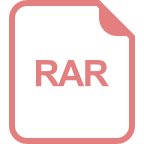
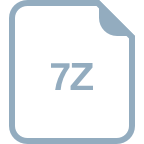
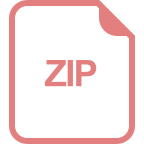
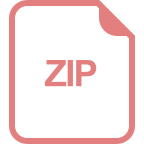
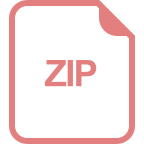
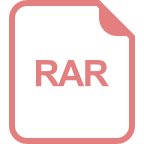
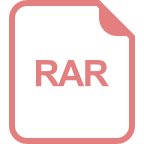
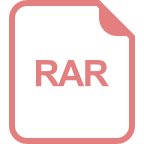
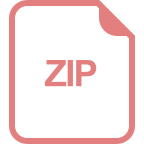
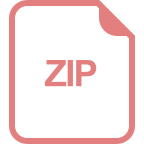
