Cplusplus定义一个定时器并且注册一个回调函数,时间到了就运行回调函数
时间: 2024-01-02 11:03:58 浏览: 25
在C++中,可以使用`std::chrono`库来实现定时器功能。下面是一个简单的实现:
```cpp
#include <iostream>
#include <chrono>
#include <thread>
#include <functional>
class Timer {
public:
Timer() : m_running(false) {}
void start(int interval, std::function<void()> callback) {
m_running = true;
std::thread([=]() {
while (m_running) {
std::this_thread::sleep_for(std::chrono::milliseconds(interval));
callback();
}
}).detach();
}
void stop() {
m_running = false;
}
private:
bool m_running;
};
int main() {
Timer timer;
timer.start(1000, []() { std::cout << "Timer callback called\n"; });
std::this_thread::sleep_for(std::chrono::seconds(5));
timer.stop();
return 0;
}
```
上述代码中,`Timer`类封装了一个定时器对象,并提供了`start`和`stop`方法来启动和停止定时器。`start`方法接受两个参数,第一个参数表示定时器的时间间隔,单位为毫秒;第二个参数是一个回调函数,表示定时器时间到了之后要执行的操作。`stop`方法用来停止定时器。
在`start`方法中,我们使用了`std::thread`来创建一个新的线程来执行定时器任务。在该线程中,我们使用`std::this_thread::sleep_for`函数来等待定时器时间间隔,然后执行回调函数。注意,在回调函数中,我们使用了Lambda表达式来定义一个匿名函数。
在`main`函数中,我们创建了一个`Timer`对象,并调用`start`方法来启动定时器。然后,我们使用`std::this_thread::sleep_for`函数来等待5秒钟,然后调用`stop`方法停止定时器。
相关推荐
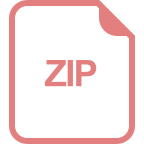
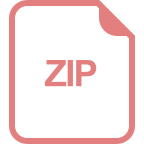














