双边滤波去噪代码matlab
时间: 2023-08-18 21:10:47 浏览: 102
以下是使用双边滤波器进行图像去噪的MATLAB代码示例:
```matlab
% 读取图像
im = imread('image.jpg');
% 设定双边滤波器参数
diameter = 10; % 滤波器直径
sigmaColor = 20; % 色彩空间标准差
sigmaSpace = 20; % 空间标准差
% 应用双边滤波器
im_filtered = imbilatfilt(im, diameter, sigmaColor, sigmaSpace);
% 显示原图和去噪后的图像
subplot(1, 2, 1);
imshow(im);
title('Original Image');
subplot(1, 2, 2);
imshow(im_filtered);
title('Filtered Image');
```
在上面的示例中,`imbilatfilt` 函数是MATLAB内置的双边滤波器函数。它需要四个参数:输入图像、滤波器直径、色彩空间标准差和空间标准差。输出是一个去噪后的图像。
相关问题
双边滤波去噪matlab代码
Sure! Here's an example of bilateral filtering denoising using MATLAB:
```matlab
% Load the noisy image
noisyImage = imread('noisy_image.png');
% Convert the image to grayscale if needed
if size(noisyImage, 3) > 1
noisyImage = rgb2gray(noisyImage);
end
% Parameters for bilateral filtering
sigmaSpatial = 2; % Spatial standard deviation
sigmaIntensity = 20; % Intensity standard deviation
% Apply bilateral filtering
filteredImage = imbilatfilt(noisyImage, sigmaSpatial, sigmaIntensity);
% Display the results
figure;
subplot(1, 2, 1);
imshow(noisyImage);
title('Noisy Image');
subplot(1, 2, 2);
imshow(filteredImage);
title('Filtered Image');
```
In this code, we first load the noisy image using the `imread` function. If the image is in color, we convert it to grayscale using the `rgb2gray` function.
Next, we define the parameters for bilateral filtering: `sigmaSpatial` controls the spatial standard deviation, which determines the size of the filtering neighborhood, and `sigmaIntensity` controls the intensity standard deviation, which determines how much the pixel values within the neighborhood affect the filtering.
We then apply bilateral filtering using the `imbilatfilt` function. This function takes the noisy image and the sigma values as input and returns the filtered image.
Finally, we display the original noisy image and the filtered image side by side using the `imshow` function and `subplot` to create a figure with two subplots.
Note: Make sure to replace `'noisy_image.png'` with the actual filename and path of your noisy image.
双边滤波去噪matlab
双边滤波是一种常用的图像去噪方法,它在能够去除噪声的同时能够保持图像的边缘信息和细节,适用于各种类型的图像。
在Matlab中,双边滤波可以通过"imbilatfilt"函数来实现。该函数的语法为:
```matlab
filtered_image = imbilatfilt(input_image, sigma_range, sigma_domain)
```
其中,input_image是待去噪的图像,sigma_range是像素值的范围标准差,通常取[10 30]之间的数值;sigma_domain是空间域标准差,通常取5左右的值。
代码示例:
```matlab
input_image = imread('noisy_image.jpg'); % 读取待去噪的图像
sigma_range = 20; % 设置像素值的范围标准差
sigma_domain = 5; % 设置空间域标准差
filtered_image = imbilatfilt(input_image, sigma_range, sigma_domain); % 双边滤波
imshow(filtered_image); % 显示去噪后的图像
```
双边滤波是通过在异质像素间加权平均的方式进行的。这种加权的方式既考虑了像素值的相似性,也考虑了像素位置的相似性,从而能够有效地去除噪声而保持图像的细节。
需要注意的是,双边滤波对处理的图像大小和噪声程度都有一定的要求,如果图像的噪声较多或图像过大,则可能需要调整参数以获得更好的去噪效果。
阅读全文
相关推荐
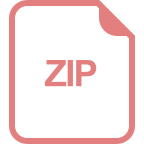
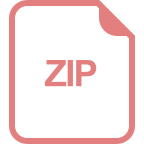
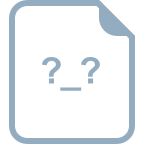



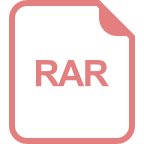
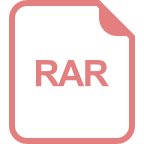
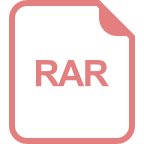

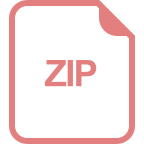
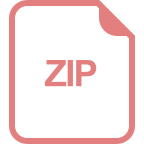
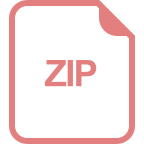
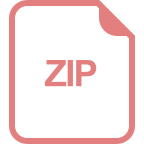
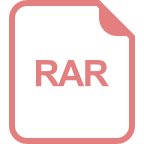
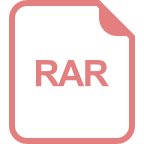