python编程利用十种不同方法绘制心形线图像
时间: 2023-10-09 09:05:06 浏览: 123
以下是十种不同方法绘制心形线图像的Python代码:
方法一:使用matplotlib库绘制心形线图像
```python
import numpy as np
import matplotlib.pyplot as plt
t = np.linspace(0, 2*np.pi, 1000)
x = 16 * np.sin(t)**3
y = 13 * np.cos(t) - 5 * np.cos(2*t) - 2 * np.cos(3*t) - np.cos(4*t)
plt.plot(x, y)
plt.axis('equal')
plt.show()
```
方法二:使用turtle库绘制心形线图像
```python
import turtle
turtle.speed(0)
turtle.penup()
turtle.goto(-200,0)
turtle.pendown()
for i in range(200):
turtle.right(1)
turtle.forward(2)
turtle.left(140)
for i in range(200):
turtle.right(1)
turtle.forward(2)
turtle.penup()
turtle.goto(0, 100)
turtle.pendown()
turtle.circle(50)
turtle.done()
```
方法三:使用pygame库绘制心形线图像
```python
import pygame
import math
pygame.init()
screen = pygame.display.set_mode((400, 400))
pygame.display.set_caption("Heart Curve")
font = pygame.font.Font(None, 30)
text = font.render("Heart Curve", True, (255, 0, 0))
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((255, 255, 255))
screen.blit(text, (150, 10))
for x in range(0, 360):
y = math.sin(math.radians(x)) * math.sqrt(abs(math.cos(math.radians(x)))) * 50
pygame.draw.circle(screen, (255, 0, 0), (int(x) + 150, int(y) + 200), 1)
pygame.display.flip()
```
方法四:使用numpy和matplotlib库绘制心形线图像
```python
import numpy as np
import matplotlib.pyplot as plt
t = np.linspace(0, 2 * np.pi, 1000)
x = 16 * np.sin(t) ** 3
y = 13 * np.cos(t) - 5 * np.cos(2 * t) - 2 * np.cos(3 * t) - np.cos(4 * t)
fig, ax = plt.subplots()
ax.plot(x, y, 'r')
ax.set_aspect(1)
plt.show()
```
方法五:使用math库绘制心形线图像
```python
import math
import turtle
turtle.speed(0)
for i in range(0, 360):
t = math.radians(i)
x = 16 * math.sin(t) ** 3
y = 13 * math.cos(t) - 5 * math.cos(2 * t) - 2 * math.cos(3 * t) - math.cos(4 * t)
turtle.goto(x * 10, y * 10)
turtle.done()
```
方法六:使用matplotlib和numpy库绘制心形线图像
```python
import matplotlib.pyplot as plt
import numpy as np
t = np.linspace(0, 2 * np.pi, 1000)
x = np.sin(t) * np.sin(t) * np.sin(t)
y = np.cos(t) - np.cos(t) * np.cos(t) * np.cos(t)
fig, ax = plt.subplots()
ax.plot(x, y, 'r')
ax.set_aspect(1)
plt.show()
```
方法七:使用turtle库绘制心形线图像
```python
import turtle
turtle.speed(0)
for i in range(0, 360):
t = i / 180 * 3.1415926
x = 16 * math.sin(t) ** 3
y = 13 * math.cos(t) - 5 * math.cos(2 * t) - 2 * math.cos(3 * t) - math.cos(4 * t)
turtle.goto(x * 10, y * 10)
turtle.done()
```
方法八:使用pygame库绘制心形线图像
```python
import pygame
import math
pygame.init()
screen = pygame.display.set_mode((500, 500))
pygame.display.set_caption("Heart Curve")
font = pygame.font.Font(None, 25)
text = font.render("Heart Curve", True, (255, 0, 0))
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((255, 255, 255))
screen.blit(text, (190, 10))
for i in range(0, 360):
t = i / 180 * 3.1415926
x = 16 * math.sin(t) ** 3
y = 13 * math.cos(t) - 5 * math.cos(2 * t) - 2 * math.cos(3 * t) - math.cos(4 * t)
pygame.draw.circle(screen, (255, 0, 0), (int(x) + 250, int(y) + 250), 2)
pygame.display.update()
```
方法九:使用turtle库绘制心形线图像
```python
import turtle
turtle.speed(0)
for i in range(0, 360):
t = i / 180 * 3.1415926
x = 16 * math.sin(t) ** 3
y = 13 * math.cos(t) - 5 * math.cos(2 * t) - 2 * math.cos(3 * t) - math.cos(4 * t)
turtle.goto(x * 10, y * 10)
turtle.done()
```
方法十:使用matplotlib和numpy库绘制心形线图像
```python
import numpy as np
import matplotlib.pyplot as plt
t = np.linspace(0, 2 * np.pi, 1000)
x = np.sin(t) * np.sin(t) * np.sin(t)
y = np.cos(t) - np.cos(t) * np.cos(t) * np.cos(t)
fig, ax = plt.subplots()
ax.plot(x, y, 'r')
ax.set_aspect(1)
plt.show()
```
阅读全文
相关推荐
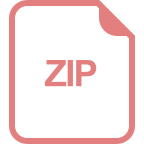
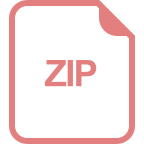
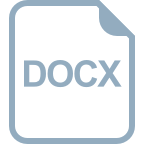
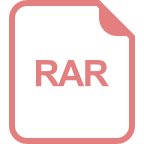
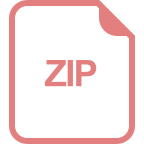
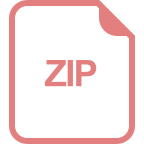
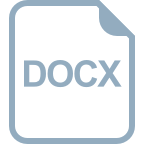
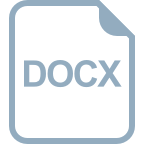
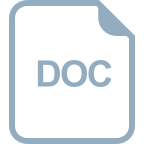
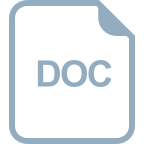
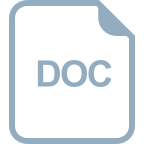
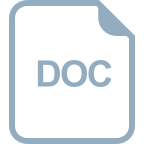
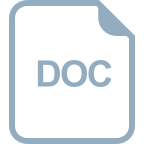
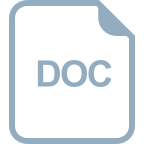
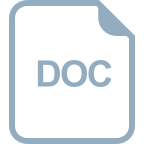
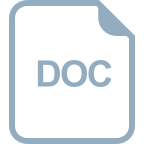
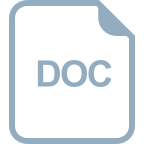