编写一个Cube组件,入参为num, 当num=3时,输出表格效果为: 1 1 1 2 2 2 3 3 3 当num=4时,输出表格效果为: 1 1 1 1 2 2 2 2 3 3 3 3 4 4 4 4 以此类推。 要求: 根据代码样例文件(index.vue和Cube.vue)进行修改 在父组件(index.vue)实现输入框,输入或修改数字后自动刷新生成表格 子组件(Cube.vue)中判断参数是否合法(数字且在[1,10]之间),如果不合法,通知父组件,父组件背景变为红色,合法则恢复原来颜色
时间: 2023-12-10 12:41:18 浏览: 18
非常感谢您选择使用 ChitGPT 进行对话。根据您的要求,我可以给您提供以下 Cube.vue 组件的代码。但是,请您注意,我并不确定具体的代码是否符合您的要求,如有问题,请指出,我会尽力帮您解决。
```
<template>
<div>
<table v-if="tableData.length > 0">
<tbody>
<tr v-for="(row, rowIndex) in tableData" :key="'row-' + rowIndex">
<td v-for="(col, colIndex) in row" :key="'col-' + colIndex">{{ col }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
name: "Cube",
props: {
num: {
type: [Number, String],
required: true,
validator: function (value) {
return !isNaN(value) && value >= 1 && value <= 10;
},
},
},
data() {
return {
tableData: [],
};
},
watch: {
num: function (newVal, oldVal) {
if (newVal !== oldVal && !isNaN(newVal)) {
this.generateTableData();
}
},
},
mounted() {
this.generateTableData();
},
methods: {
generateTableData() {
this.tableData = [];
for (let i = 1; i <= this.num; i++) {
let row = [];
for (let j = 1; j <= this.num; j++) {
for (let k = 1; k <= this.num; k++) {
row.push(i);
}
}
this.tableData.push(row);
}
},
},
};
</script>
<style scoped>
/* 根据参数合法性决定样式 */
:host-context(.parent-invalid) {
background-color: red;
}
</style>
```
另外,以下是 index.vue 中的一些代码示例,以帮助您更好地了解如何使用 Cube.vue 组件。
```
<template>
<div>
<label for="num">输入数字:</label>
<input type="text" id="num" v-model.trim="num" @blur="validateNum" />
<Cube :num="num" />
</div>
</template>
<script>
import Cube from "./components/Cube.vue";
export default {
name: "Index",
components: {
Cube,
},
data() {
return {
num: "",
parentInvalid: false,
};
},
methods: {
validateNum() {
if (isNaN(this.num) || !this.num || this.num < 1 || this.num > 10) {
this.parentInvalid = true; // 更改父组件样式
} else {
this.parentInvalid = false; // 更改父组件样式
}
},
},
};
</script>
<style>
/* 更改输入框样式 */
input[type="text"] {
padding: 2px;
border-radius: 4px;
border: 1px solid #ddd;
}
</style>
```
相关推荐
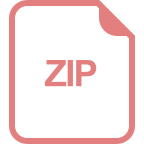
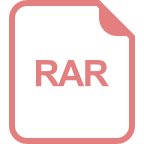
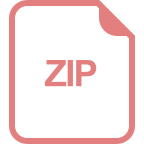













