1.在E盘根目录下创建文件名为iotest1.txt其内容为 inputstream. 创建文件输入流将iotest1txt文件内容读出,并显示其内容。读出的方式要求如下: ①一次只读一个字节,编写程序1-1.②一次读多个字节,编写程序 ③使用缓冲区输入流,一次读多个字节,编写程序
时间: 2024-02-29 17:51:43 浏览: 95
好的,我会为您回答这个问题。以下是三种不同的方式来读取文件"E:/iotest1.txt"的内容:
1. 一次只读一个字节,编写程序1-1:
```java
import java.io.*;
public class ReadFileOneByte {
public static void main(String[] args) {
File file = new File("E:/iotest1.txt");
try {
FileInputStream fis = new FileInputStream(file);
int content;
while ((content = fis.read()) != -1) {
System.out.print((char) content);
}
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
2. 一次读多个字节,编写程序:
```java
import java.io.*;
public class ReadFileMultipleBytes {
public static void main(String[] args) {
File file = new File("E:/iotest1.txt");
try {
FileInputStream fis = new FileInputStream(file);
byte[] contentBytes = new byte[1024];
int bytesRead;
while ((bytesRead = fis.read(contentBytes)) != -1) {
System.out.print(new String(contentBytes, 0, bytesRead));
}
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
3. 使用缓冲区输入流,一次读多个字节,编写程序:
```java
import java.io.*;
public class ReadFileBuffered {
public static void main(String[] args) {
File file = new File("E:/iotest1.txt");
try {
FileInputStream fis = new FileInputStream(file);
BufferedInputStream bis = new BufferedInputStream(fis);
byte[] contentBytes = new byte[1024];
int bytesRead;
while ((bytesRead = bis.read(contentBytes)) != -1) {
System.out.print(new String(contentBytes, 0, bytesRead));
}
bis.close();
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
请注意,这三种方法都可以读取文件的内容,但是它们的效率和性能有所不同。一次只读一个字节的方法可能会非常慢,而使用缓冲区输入流可以大大提高读取的速度。
阅读全文
相关推荐
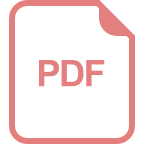
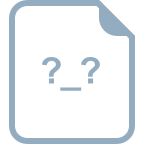
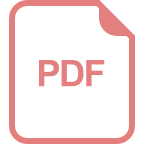















