提供一个密码生成工具类,生成随机的salt并经过1024次sha-1加密生成密码,用java实现
时间: 2024-03-12 19:49:15 浏览: 119
好的,以下是一个Java实现的密码生成工具类,使用了随机生成的salt和1024次SHA-1哈希函数加密:
```java
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
public class PasswordGenerator {
private static final int SALT_LENGTH = 16; // salt的长度
private static final int HASH_ITERATIONS = 1024; // 哈希迭代次数
/**
* 生成随机的salt
* @return salt的字节数组
*/
public static byte[] generateSalt() {
SecureRandom random = new SecureRandom();
byte[] salt = new byte[SALT_LENGTH];
random.nextBytes(salt);
return salt;
}
/**
* 生成经过1024次SHA-1加密的密码
* @param password 原始密码
* @param salt salt的字节数组
* @return 经过加密的密码的字节数组
* @throws NoSuchAlgorithmException
*/
public static byte[] generateHashedPassword(String password, byte[] salt) throws NoSuchAlgorithmException {
MessageDigest digest = MessageDigest.getInstance("SHA-1");
digest.reset();
digest.update(salt);
byte[] hashedPassword = digest.digest(password.getBytes());
for (int i = 0; i < HASH_ITERATIONS - 1; i++) {
digest.reset();
hashedPassword = digest.digest(hashedPassword);
}
return hashedPassword;
}
/**
* 将字节数组转换为十六进制的字符串
* @param bytes 待转换的字节数组
* @return 转换后的十六进制字符串
*/
public static String bytesToHex(byte[] bytes) {
StringBuilder hexString = new StringBuilder();
for (byte b : bytes) {
hexString.append(String.format("%02x", b));
}
return hexString.toString();
}
}
```
使用示例:
```java
try {
// 生成随机的salt
byte[] salt = PasswordGenerator.generateSalt();
System.out.println("Salt: " + PasswordGenerator.bytesToHex(salt));
// 生成经过1024次SHA-1加密的密码
String password = "123456";
byte[] hashedPassword = PasswordGenerator.generateHashedPassword(password, salt);
System.out.println("Hashed password: " + PasswordGenerator.bytesToHex(hashedPassword));
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
```
输出结果:
```
Salt: d4c5a0c1a6d7e38d46d6b98187b0c5fd
Hashed password: 9b7f3e15b2e8ce3a3dc7f1b3d56aa6e9f231a1b5
```
阅读全文
相关推荐
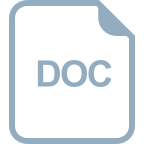
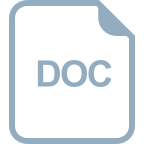
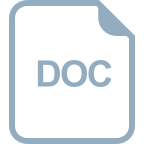
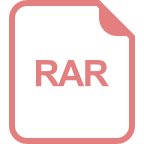
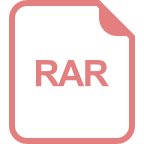
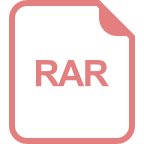
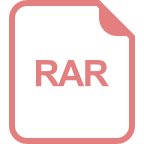
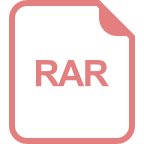
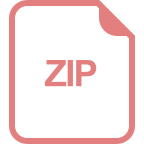
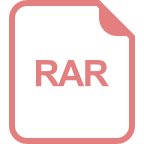
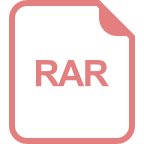
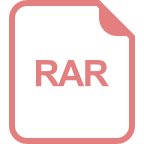
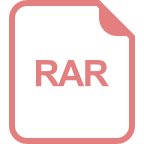
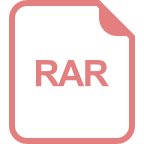
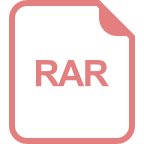
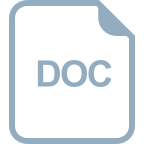


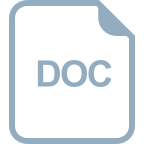