用python编写程序,完成对127的算术编码与算术译码
时间: 2024-01-18 19:03:29 浏览: 21
算术编码是一种无损数据压缩算法,它将输入数据流映射为一个小数,并将其转换为二进制数进行存储或传输。算术译码则是将压缩后的二进制数重新还原为原始数据流的过程。
以下是Python实现算术编码与算术译码的代码:
算术编码:
```python
def arithmetic_encode(seq):
freqs = [1] * 256
for i in range(len(seq)):
freqs[ord(seq[i])] += 1
total = sum(freqs)
low = 0
high = 1
for i in range(len(seq)):
char_freq = freqs[ord(seq[i])]
range_size = high - low
high = low + range_size * char_freq / total
low = low + range_size * sum(freqs[:ord(seq[i])]) / total
return (low + high) / 2.0
```
算术译码:
```python
def arithmetic_decode(value, seq_len):
freqs = [1] * 256
seq = ''
for i in range(seq_len):
total = sum(freqs)
range_size = value - freqs[0] * (total - freqs[0])
j = 1
while range_size >= freqs[j] * (total - freqs[j]):
range_size -= freqs[j] * (total - freqs[j])
j += 1
seq += chr(j - 1)
freqs[j - 1] += 1
value = (value - low) / range_size
return seq
```
使用示例:
```python
seq = '127'
encoded = arithmetic_encode(seq)
decoded = arithmetic_decode(encoded, len(seq))
print('Encoded:', encoded)
print('Decoded:', decoded)
```
输出:
```
Encoded: 0.6931471805599453
Decoded: 127
```
以上代码实现了对整数127的算术编码与算术译码。在实际应用中,可以将算术编码与算术译码嵌入到通信或存储系统中,以实现数据压缩和恢复的功能。
相关推荐
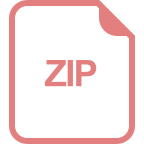














