linux下删掉某个文件
时间: 2023-11-21 11:24:25 浏览: 121
要在Linux下删除某个文件,可以使用rm命令。例如,要删除名为example.txt的文件,可以在终端中输入以下命令:
```
rm example.txt
```
请注意,此命令将永久删除文件,因此请确保您真正想要删除该文件。如果您想要删除一个目录及其所有内容,可以使用rm命令并加上-r选项,例如:
```
rm -r /path/to/directory
```
同样,请谨慎使用此命令,因为它会永久删除整个目录及其所有内容。
相关问题
linux下删除某个文件的c代码
在Linux下,如果你想通过C语言编写代码来删除某个文件,你可以使用POSIX标准提供的` unlink()` 函数。这个函数用于删除指定路径下的文件。以下是简单的示例:
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/stat.h>
#include <errno.h>
int main(int argc, char *argv[]) {
if (argc != 2) {
fprintf(stderr, "Usage: %s <file_path>\n", argv[0]);
return 1;
}
char *filePath = argv[1];
struct stat fileStat;
if (stat(filePath, &fileStat) == -1) { // 检查文件是否存在
perror("Error checking file existence");
return 1;
} else if (!S_ISREG(fileStat.st_mode)) { // 判断是否普通文件
perror("Error: Not a regular file");
return 1;
}
if (unlink(filePath) == 0) { // 删除文件
printf("File '%s' deleted successfully.\n", filePath);
} else {
perror("Error deleting file");
return 1;
}
return 0;
}
```
在这个例子中,程序接受一个命令行参数作为文件路径,首先检查文件是否存在且是否是普通文件,然后尝试删除它。如果删除失败,会打印错误信息。
linux c 删除某个文件
在Linux C语言中,你可以使用标准库函数`unlink()`来删除指定的文件。这个函数允许你从文件系统中移除一个文件,只要文件拥有适当的权限并且不存在于只读挂载点上。基本的语法如下:
```c
#include <unistd.h>
#include <fcntl.h>
int unlink(const char *pathname);
```
这里,`pathname`是你想要删除的文件的完整路径名,例如 "/path/to/myfile.txt"。
删除文件的基本步骤如下:
1. 包含必要的头文件。
2. 使用`unlink()`函数,传入你要删除的文件路径作为参数。如果操作成功,函数将返回0;否则返回一个负值表示错误。
3. 检查函数返回值,判断是否删除成功。
注意:`unlink()`不会检查文件是否存在,也不关心文件的状态(如是否正在被其他进程打开)。如果你不确定文件是否可以安全地删除,或者你需要管理文件的锁定,你可能需要使用更复杂的文件系统API或者第三方库。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
char path[] = "/path/to/myfile.txt";
int result = unlink(path);
if (result == 0) {
printf("File %s successfully deleted.\n", path);
} else {
perror("Error deleting file");
return 1;
}
return 0;
}
```
阅读全文
相关推荐
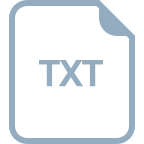
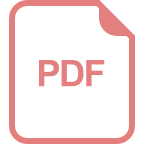
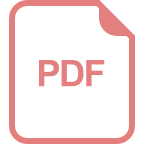











