帮我写一个能在电脑上运行的数独小游戏
时间: 2023-11-24 09:06:02 浏览: 190
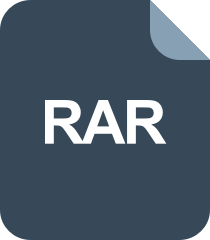
数独小游戏
好的,我可以为您提供一个Python的数独小游戏,您可以在电脑上运行。
代码如下:
```python
import pygame
import sudoku_generator
import time
# 初始化pygame
pygame.init()
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GRAY = (128, 128, 128)
LIGHT_BLUE = (96, 216, 232)
LIGHT_YELLOW = (255, 244, 79)
# 设置屏幕大小和标题
size = (540, 600)
screen = pygame.display.set_mode(size)
pygame.display.set_caption("数独小游戏")
# 设定字体
font = pygame.font.SysFont('Arial', 30)
# 定义数独生成器
sudoku = sudoku_generator.SudokuGenerator()
# 生成数独谜题和答案
sudoku.generate()
puzzle = sudoku.puzzle
solution = sudoku.solution
# 定义方格的边长
square_size = 60
# 定义数独面板的位置和大小
board_x = (size[0] - square_size * 9) / 2
board_y = (size[1] - square_size * 9) / 2
board_width = square_size * 9
board_height = square_size * 9
# 定义选中的方格的位置和大小
selected_x = None
selected_y = None
selected_width = square_size
selected_height = square_size
# 定义游戏是否结束
game_over = False
# 定义数独面板的二维数组
board = [[0 for x in range(9)] for y in range(9)]
# 将谜题复制到数独面板中
for i in range(9):
for j in range(9):
board[i][j] = puzzle[i][j]
# 定义数独面板的函数
def draw_board():
for i in range(9):
for j in range(9):
# 绘制方格
pygame.draw.rect(screen, BLACK, [board_x + square_size * j, board_y + square_size * i, square_size, square_size], 2)
# 绘制数字
if board[i][j] != 0:
text = font.render(str(board[i][j]), True, BLACK)
text_rect = text.get_rect(center=(board_x + square_size * j + square_size / 2, board_y + square_size * i + square_size / 2))
screen.blit(text, text_rect)
# 定义选中方格的函数
def draw_selected(x, y):
pygame.draw.rect(screen, LIGHT_YELLOW, [board_x + square_size * x, board_y + square_size * y, selected_width, selected_height], 3)
# 定义检查数独是否已完成的函数
def check_board():
for i in range(9):
for j in range(9):
if board[i][j] == 0:
return False
return True
# 游戏循环
clock = pygame.time.Clock()
while not game_over:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.MOUSEBUTTONDOWN:
# 获取鼠标点击的位置
pos = pygame.mouse.get_pos()
# 判断是否点击了数独面板内的方格
if board_x <= pos[0] <= board_x + board_width and board_y <= pos[1] <= board_y + board_height:
selected_x = int((pos[0] - board_x) / square_size)
selected_y = int((pos[1] - board_y) / square_size)
# 如果点击的方格是谜题中的空格,选中该方格
if puzzle[selected_y][selected_x] == 0:
draw_selected(selected_x, selected_y)
else:
selected_x = None
selected_y = None
elif event.type == pygame.KEYDOWN and selected_x is not None and selected_y is not None:
# 如果按下数字键,将数字填入选中的方格
if event.unicode.isdigit() and puzzle[selected_y][selected_x] == 0:
board[selected_y][selected_x] = int(event.unicode)
selected_x = None
selected_y = None
# 如果按下删除键,将选中的方格清空
elif event.key == pygame.K_BACKSPACE or event.key == pygame.K_DELETE:
board[selected_y][selected_x] = 0
selected_x = None
selected_y = None
# 绘制屏幕
screen.fill(WHITE)
draw_board()
if selected_x is not None and selected_y is not None:
draw_selected(selected_x, selected_y)
# 检查数独是否已完成
if check_board():
# 如果已完成,绘制背景色并显示"恭喜你,完成了!"的提示
pygame.draw.rect(screen, LIGHT_BLUE, [board_x, board_y, board_width, board_height])
text = font.render("恭喜你,完成了!", True, BLACK)
text_rect = text.get_rect(center=(size[0] / 2, board_y - 50))
screen.blit(text, text_rect)
# 更新屏幕
pygame.display.flip()
# 控制帧率
clock.tick(60)
# 退出游戏
pygame.quit()
```
这个数独小游戏使用了Pygame库,可以在终端中运行。您可以将代码保存为.py文件,然后在终端中运行。
运行后,您可以使用鼠标点击数独面板中的方格,然后按数字键将数字填入方格中。如果需要清空已填入的数字,可以按下删除键或退格键。当您完成数独后,屏幕会显示"恭喜你,完成了!"的提示。
希望这个数独小游戏能够带给您一些乐趣!
阅读全文
相关推荐
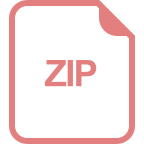
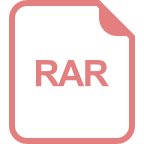
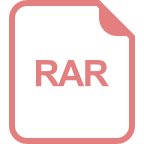
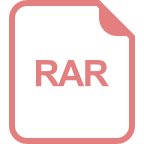
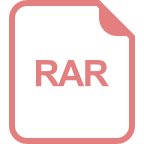
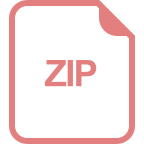
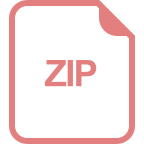
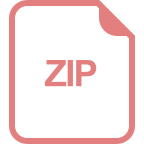
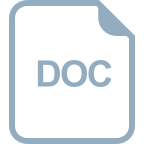
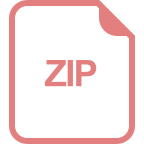
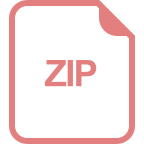
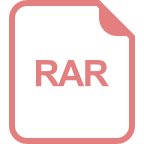
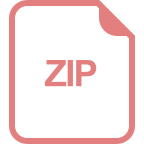
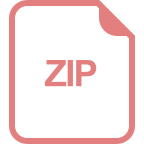
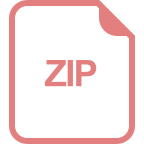
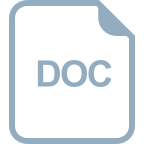