Qt中不使用opengl如何实现鼠标移动控制模型旋转功能,已知视图矩阵viewMatrix,投影矩阵prijectionMatrix,模型矩阵modelMatrix和相机旋转(rx,ry,rz)和相机位置(tx,ty,tz)
时间: 2024-03-28 07:42:22 浏览: 112
要实现鼠标移动控制模型旋转功能,需要通过计算出旋转矩阵,来更新模型矩阵。
首先,需要根据鼠标移动的距离计算出旋转角度,可以使用以下代码:
```C++
float dx = mouseCurrentX - mousePreviousX;
float dy = mouseCurrentY - mousePreviousY;
float angleX = dx / windowWidth * 2;
float angleY = dy / windowHeight * 2;
```
其中,mouseCurrentX和mouseCurrentY是当前鼠标的位置,mousePreviousX和mousePreviousY是上一次鼠标的位置,windowWidth和windowHeight是窗口的宽度和高度。
接下来,需要根据相机的旋转角度和位置,以及计算出的旋转角度,计算出新的视图矩阵。可以使用以下代码:
```C++
float cosX = cos(angleX);
float sinX = sin(angleX);
float cosY = cos(angleY);
float sinY = sin(angleY);
// 计算相机的旋转矩阵
QMatrix4x4 cameraRotation;
cameraRotation.setToIdentity();
cameraRotation.rotate(rx, 1, 0, 0);
cameraRotation.rotate(ry, 0, 1, 0);
cameraRotation.rotate(rz, 0, 0, 1);
// 计算相机的平移矩阵
QMatrix4x4 cameraTranslation;
cameraTranslation.setToIdentity();
cameraTranslation.translate(-tx, -ty, -tz);
// 计算旋转矩阵
QMatrix4x4 rotation;
rotation.setToIdentity();
rotation.rotate(angleY, cosX, 0, -sinX);
rotation.rotate(angleX, 0, 1, 0);
// 计算新的视图矩阵
viewMatrix = rotation * cameraRotation * cameraTranslation;
```
其中,rx、ry、rz是相机的旋转角度,tx、ty、tz是相机的位置。
最后,将视图矩阵、投影矩阵和模型矩阵相乘,得到最终的变换矩阵:
```C++
QMatrix4x4 transformMatrix = prijectionMatrix * viewMatrix * modelMatrix;
```
阅读全文
相关推荐
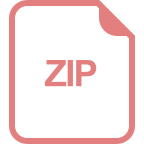
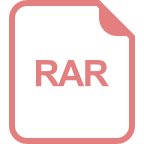
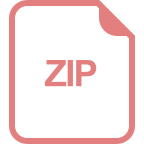
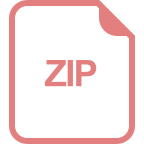
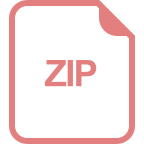
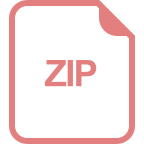
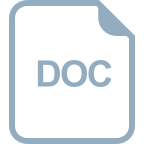
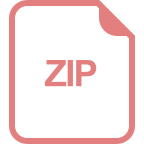
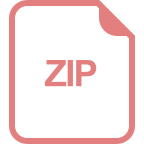
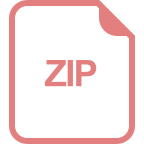
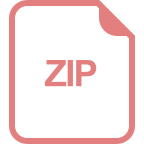
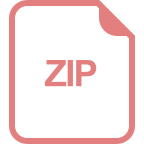
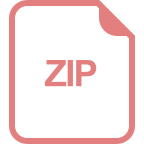