用C语言查找链表最后节点
时间: 2023-07-10 08:32:40 浏览: 209
好的,以下是用 C 语言实现查找链表最后节点的示例代码:
```c
struct Node {
int data;
struct Node* next;
};
struct Node* getLastNode(struct Node* head) {
if (head == NULL) {
return NULL;
}
struct Node* current = head;
while (current->next != NULL) {
current = current->next;
}
return current;
}
```
其中,Node 是链表节点的结构体,包含一个数据成员和一个指向下一个节点的指针成员。函数 getLastNode 接受一个头节点指针作为参数,返回最后一个节点的指针。函数首先检查头节点是否为 NULL,如果是,则返回 NULL,否则从头节点开始遍历链表,直到找到最后一个节点,并返回该节点的指针。
示例用法:
```c
int main() {
// 创建链表
struct Node* head = malloc(sizeof(struct Node));
head->data = 1;
head->next = NULL;
struct Node* node1 = malloc(sizeof(struct Node));
node1->data = 2;
node1->next = NULL;
head->next = node1;
struct Node* node2 = malloc(sizeof(struct Node));
node2->data = 3;
node2->next = NULL;
node1->next = node2;
// 查找最后一个节点
struct Node* lastNode = getLastNode(head);
printf("Last node data: %d\n", lastNode->data);
// 释放链表内存
free(node2);
free(node1);
free(head);
return 0;
}
```
阅读全文
相关推荐
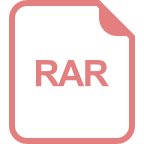






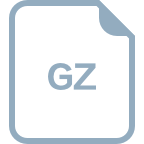








