Win32API COM读取Excel表格源码 封装工具类
时间: 2023-11-28 14:50:25 浏览: 95
以下是一个简单的 Win32API COM 读取 Excel 表格的工具类封装源码:
```c++
#include <Windows.h>
#include <Ole2.h>
#include <OleAuto.h>
#include <iostream>
#include <string>
using namespace std;
class ExcelReader
{
public:
ExcelReader();
~ExcelReader();
bool Open(const char* filename);
void Close();
bool ReadCell(int row, int col, string& str);
private:
bool Init();
bool Uninit();
private:
HRESULT m_hr;
IDispatch* m_pExcelApp;
_Application* m_pExcel;
Workbooks* m_pBooks;
_Workbook* m_pBook;
Worksheets* m_pSheets;
_Worksheet* m_pSheet;
};
ExcelReader::ExcelReader()
:m_hr(S_OK), m_pExcelApp(NULL), m_pExcel(NULL), m_pBooks(NULL), m_pBook(NULL), m_pSheets(NULL), m_pSheet(NULL)
{
Init();
}
ExcelReader::~ExcelReader()
{
Uninit();
}
bool ExcelReader::Init()
{
m_hr = CoInitialize(NULL);
if (FAILED(m_hr))
{
return false;
}
m_hr = CoCreateInstance(CLSID_Application, NULL, CLSCTX_LOCAL_SERVER, IID_IDispatch, (void**)&m_pExcelApp);
if (FAILED(m_hr))
{
return false;
}
m_pExcel = (_Application*)m_pExcelApp;
m_pBooks = m_pExcel->Workbooks;
return true;
}
bool ExcelReader::Uninit()
{
if (m_pSheet != NULL)
{
m_pSheet->Release();
m_pSheet = NULL;
}
if (m_pSheets != NULL)
{
m_pSheets->Release();
m_pSheets = NULL;
}
if (m_pBook != NULL)
{
m_pBook->Close(VARIANT_TRUE);
m_pBook->Release();
m_pBook = NULL;
}
if (m_pBooks != NULL)
{
m_pBooks->Release();
m_pBooks = NULL;
}
if (m_pExcel != NULL)
{
m_pExcel->Quit();
m_pExcel = NULL;
}
if (m_pExcelApp != NULL)
{
m_pExcelApp->Release();
m_pExcelApp = NULL;
}
CoUninitialize();
return true;
}
bool ExcelReader::Open(const char* filename)
{
if (m_pExcel == NULL)
{
return false;
}
m_pBook = m_pBooks->Open(_bstr_t(filename));
if (m_pBook == NULL)
{
return false;
}
m_pSheets = m_pBook->Worksheets;
m_pSheet = (_Worksheet*)m_pSheets->Item(1);
if (m_pSheet == NULL)
{
return false;
}
return true;
}
void ExcelReader::Close()
{
Uninit();
}
bool ExcelReader::ReadCell(int row, int col, string& str)
{
if (m_pSheet == NULL)
{
return false;
}
_variant_t val;
val = m_pSheet->Cells->Item[row][col]->Value;
if (val.vt == VT_NULL)
{
return false;
}
_bstr_t bstr = val;
str = (char*)bstr;
return true;
}
```
使用方法:
```c++
ExcelReader reader;
if (!reader.Open("test.xlsx"))
{
cout << "Open file failed." << endl;
return 0;
}
string cell_value;
if (reader.ReadCell(1, 1, cell_value))
{
cout << "Cell value: " << cell_value << endl;
}
else
{
cout << "Read cell failed." << endl;
}
reader.Close();
```
注意,需要在代码中引入 `Ole2.h`、`OleAuto.h` 头文件,并且在工程链接时需要添加 `Ole32.lib`、`OleAut32.lib` 库文件。
阅读全文
相关推荐
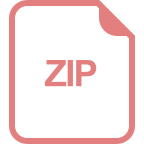
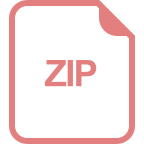
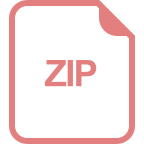
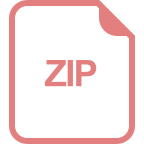
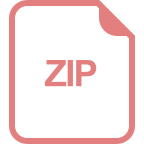
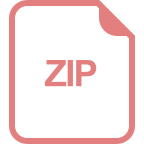
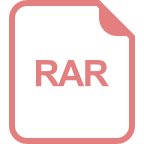
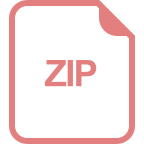
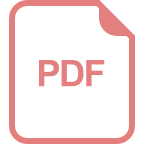
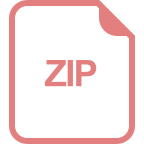
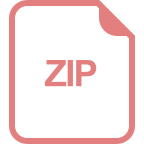
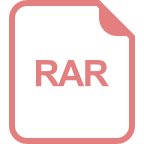
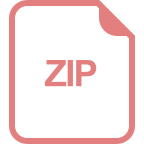
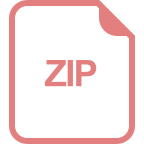
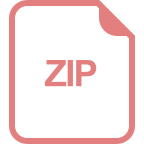