帮我写一个C语言的贪吃蛇排线
时间: 2023-04-08 15:04:37 浏览: 65
好的,我可以回答这个问题。以下是一个简单的C语言贪吃蛇排线的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 30
#define HEIGHT 20
int snake[100][2]; // 蛇的坐标数组
int length = 3; // 蛇的长度
int direction = 1; // 蛇的方向,1表示向右,2表示向下,3表示向左,4表示向上
int food_x, food_y; // 食物的坐标
void gotoxy(int x, int y) // 光标移动函数
{
COORD pos = {x, y};
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), pos);
}
void draw_map() // 绘制地图函数
{
int i, j;
for (i = 0; i <= HEIGHT + 1; i++) {
for (j = 0; j <= WIDTH + 1; j++) {
if (i == 0 || i == HEIGHT + 1 || j == 0 || j == WIDTH + 1) {
printf("#");
} else {
printf(" ");
}
}
printf("\n");
}
}
void draw_snake() // 绘制蛇函数
{
int i;
for (i = 0; i < length; i++) {
gotoxy(snake[i][0], snake[i][1]);
printf("*");
}
}
void generate_food() // 生成食物函数
{
srand((unsigned)time(NULL));
food_x = rand() % WIDTH + 1;
food_y = rand() % HEIGHT + 1;
gotoxy(food_x, food_y);
printf("@");
}
void move_snake() // 移动蛇函数
{
int i;
for (i = length - 1; i > 0; i--) {
snake[i][0] = snake[i - 1][0];
snake[i][1] = snake[i - 1][1];
}
switch (direction) {
case 1:
snake[0][0]++;
break;
case 2:
snake[0][1]++;
break;
case 3:
snake[0][0]--;
break;
case 4:
snake[0][1]--;
break;
}
}
int check_collision() // 检查碰撞函数
{
if (snake[0][0] == 0 || snake[0][0] == WIDTH + 1 || snake[0][1] == 0 || snake[0][1] == HEIGHT + 1) {
return 1; // 撞墙了
}
int i;
for (i = 1; i < length; i++) {
if (snake[0][0] == snake[i][0] && snake[0][1] == snake[i][1]) {
return 1; // 撞到自己了
}
}
if (snake[0][0] == food_x && snake[0][1] == food_y) {
length++; // 吃到食物,蛇变长
generate_food(); // 重新生成食物
}
return 0;
}
int main()
{
int i;
for (i = 0; i < length; i++) {
snake[i][0] = WIDTH / 2 + i;
snake[i][1] = HEIGHT / 2;
}
draw_map();
draw_snake();
generate_food();
while (1) {
if (_kbhit()) { // 检测键盘输入
char ch = _getch();
if (ch == 'w' && direction != 2) {
direction = 4;
}
if (ch == 's' && direction != 4) {
direction = 2;
}
if (ch == 'a' && direction != 1) {
direction = 3;
}
if (ch == 'd' && direction != 3) {
direction = 1;
}
}
move_snake();
if (check_collision()) {
gotoxy(WIDTH / 2 - 4, HEIGHT / 2);
printf("Game Over!");
break;
}
draw_snake();
Sleep(100); // 控制蛇的速度
}
return 0;
}
```
相关推荐
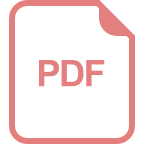
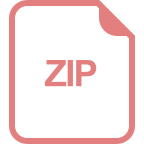














