typeScript笔试题
时间: 2023-08-01 13:14:07 浏览: 42
好的,以下是一些 TypeScript 笔试题:
1. TypeScript 中的接口是什么?请举例说明。
答:接口(Interface)是 TypeScript 中的一种类型,用于描述对象的形状。它定义了一个对象应该有哪些属性和方法,但不包含实现细节。例如:
```
interface Person {
name: string;
age: number;
sayHello(): void;
}
const alice: Person = {
name: "Alice",
age: 30,
sayHello() {
console.log(`Hello, my name is ${this.name}.`);
}
};
```
2. TypeScript 中的枚举是什么?请举例说明。
答:枚举(Enum)是 TypeScript 中的一种类型,用于定义一组有限的命名常量。例如:
```
enum Color {
Red,
Green,
Blue
}
const color: Color = Color.Green;
console.log(color); // 输出 1
```
在上面的例子中,枚举 Color 定义了三个常量 Red、Green 和 Blue,它们的值分别为 0、1 和 2。可以通过枚举的名称或值来访问它们。
3. TypeScript 中的泛型是什么?请举例说明。
答:泛型(Generics)是 TypeScript 中的一种机制,用于编写可重用的代码。它允许在定义函数、类或接口时使用类型参数,以便在使用时动态地指定类型。例如:
```
function identity<T>(arg: T): T {
return arg;
}
const result1 = identity("hello"); // 类型推断为 string
const result2 = identity<number>(42); // 指定类型为 number
```
在上面的例子中,函数 identity 接受一个类型为 T 的参数,并返回一个类型为 T 的值。使用 <T> 表示类型参数,并在调用时通过传递的参数类型来动态指定 T 的类型。
4. TypeScript 中的类是什么?请举例说明。
答:类(Class)是 TypeScript 中的一种机制,用于创建对象的模板。它包含了属性和方法的定义,可以通过 new 关键字来实例化。例如:
```
class Person {
name: string;
age: number;
constructor(name: string, age: number) {
this.name = name;
this.age = age;
}
sayHello() {
console.log(`Hello, my name is ${this.name}.`);
}
}
const alice = new Person("Alice", 30);
alice.sayHello();
```
在上面的例子中,类 Person 定义了两个属性 name 和 age,一个构造函数和一个方法 sayHello。可以通过 new 关键字来创建一个 Person 类的实例,并调用它的方法 sayHello。
相关推荐
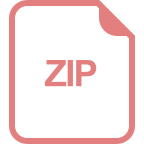
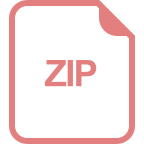
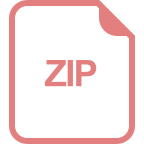











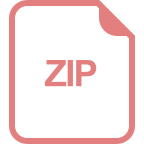
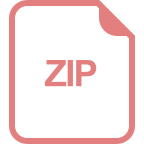
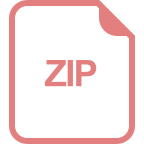
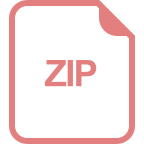