vue 调用node.js 字进程案例
时间: 2023-07-09 10:10:37 浏览: 113
在 Vue 中调用 Node.js 子进程可以使用 Node.js 的 `child_process` 模块,具体操作步骤如下:
1. 在 Vue 项目根目录下,新建一个 `server.js` 文件,用于启动一个 Node.js 服务,代码如下:
```javascript
const http = require('http');
const { spawn } = require('child_process');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
// 调用子进程执行 shell 命令
const child = spawn('ls', ['-lh', '/usr']);
let data = '';
child.stdout.on('data', (chunk) => {
data += chunk;
});
child.stdout.on('end', () => {
res.end(data);
});
});
server.listen(3000, () => {
console.log('Server started on port 3000');
});
```
上面的代码中,我们启动了一个 HTTP 服务,并在其中调用了子进程执行 `ls -lh /usr` 命令,并将结果返回给客户端。
2. 在 Vue 组件中,通过 `axios` 或者其他 HTTP 客户端库,向刚才启动的 Node.js 服务发送请求,代码如下:
```javascript
methods: {
async callChildProcess() {
const response = await axios.get('http://localhost:3000');
console.log(response.data);
},
},
```
上面的代码中,我们在 Vue 组件的 `callChildProcess` 方法中使用了 `axios` 库发送了一个 GET 请求到 `http://localhost:3000`,并打印了响应数据。
3. 在 Vue 组件中,将 `callChildProcess` 方法绑定到某个按钮的点击事件上,实现用户点击按钮时调用子进程,代码如下:
```html
<template>
<div>
<button @click="callChildProcess">Call Child Process</button>
</div>
</template>
<script>
import axios from 'axios';
export default {
methods: {
async callChildProcess() {
const response = await axios.get('http://localhost:3000');
console.log(response.data);
},
},
};
</script>
```
上面的代码中,我们在模板中添加了一个按钮,并将 `callChildProcess` 方法绑定到按钮的点击事件上。
以上就是在 Vue 中调用 Node.js 子进程的基本操作步骤。需要注意的是,在实际开发中,我们需要根据具体的业务需求来修改上面的代码。
阅读全文
相关推荐
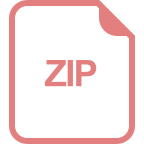
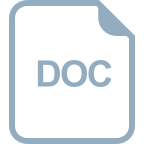
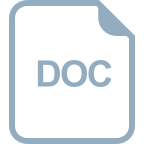
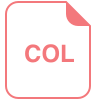
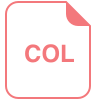
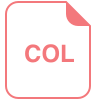
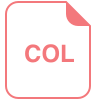
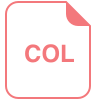
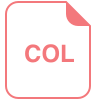
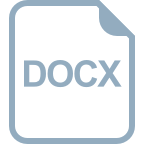
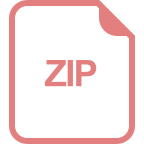
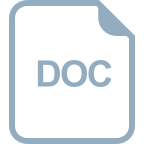
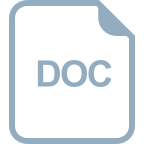
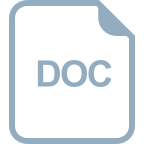
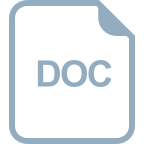
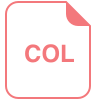
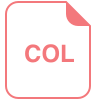