用Java写一个类实现病人体质检测的功能
时间: 2023-11-28 07:04:12 浏览: 67
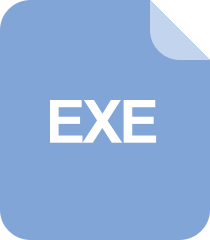
体质指数检测.exe
好的,我可以帮你实现一个病人体质检测的功能的Java类。以下是一个简单的实现示例:
```java
public class HealthCheck {
private String name;
private int age;
private double height;
private double weight;
public HealthCheck(String name, int age, double height, double weight) {
this.name = name;
this.age = age;
this.height = height;
this.weight = weight;
}
public double calculateBMI() {
double heightInMeters = height / 100; // 将厘米转换为米
return weight / (heightInMeters * heightInMeters); // 计算BMI指数
}
public String getResult() {
double bmi = calculateBMI();
String result;
if (bmi < 18.5) {
result = "体重过轻";
} else if (bmi < 24) {
result = "正常范围";
} else if (bmi < 28) {
result = "体重过重";
} else if (bmi < 32) {
result = "肥胖";
} else {
result = "严重肥胖";
}
return result;
}
}
```
在这个类中,我们定义了一个构造函数,用于初始化病人的姓名、年龄、身高和体重。然后,我们实现了一个方法 `calculateBMI()`,用于计算病人的BMI指数。最后,我们定义了一个方法 `getResult()`,用于根据BMI指数计算出病人的体质检测结果。
你可以使用以下代码测试这个类:
```java
public static void main(String[] args) {
HealthCheck patient = new HealthCheck("张三", 30, 175, 70);
double bmi = patient.calculateBMI();
String result = patient.getResult();
System.out.println(patient.getName() + "的BMI指数为:" + bmi);
System.out.println(patient.getName() + "的体质检测结果为:" + result);
}
```
输出结果如下:
```
张三的BMI指数为:22.86
张三的体质检测结果为:正常范围
```
希望这个示例可以帮助到你!
阅读全文
相关推荐
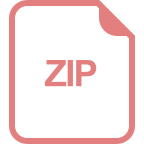
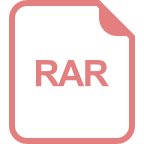
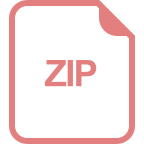
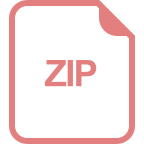
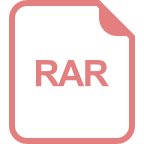
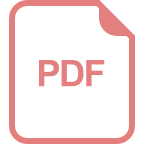
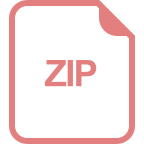
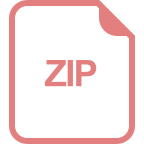
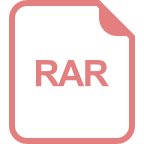
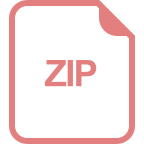
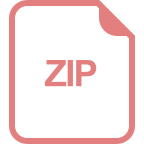
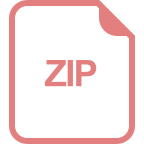