java实现请假功能代码
时间: 2023-08-30 16:06:31 浏览: 298
请假功能的实现可以分为前端和后端两部分,前端部分主要是页面设计和用户输入的数据收集,后端部分则主要是数据处理和业务逻辑实现。下面是一个简单的Java实现请假功能的示例代码,其中采用了Spring Boot框架和MySQL数据库:
1. 创建请假申请表
首先我们需要在MySQL数据库中创建一个请假申请表,用来存储请假申请的相关信息,表结构如下:
```sql
CREATE TABLE `leave_application` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(50) NOT NULL COMMENT '请假人姓名',
`start_time` datetime NOT NULL COMMENT '请假开始时间',
`end_time` datetime NOT NULL COMMENT '请假结束时间',
`reason` varchar(500) NOT NULL COMMENT '请假原因',
`status` tinyint(4) NOT NULL DEFAULT '0' COMMENT '审批状态,0表示未审批,1表示已通过,2表示未通过',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COMMENT='请假申请表';
```
2. 创建Java实体类
在Java代码中,我们需要定义一个LeaveApplication类来对应请假申请表中的一条记录,代码如下:
```java
import java.time.LocalDateTime;
public class LeaveApplication {
private Integer id; // 请假申请ID
private String name; // 请假人姓名
private LocalDateTime startTime; // 请假开始时间
private LocalDateTime endTime; // 请假结束时间
private String reason; // 请假原因
private Integer status; // 审批状态,0表示未审批,1表示已通过,2表示未通过
// 省略getter和setter方法
}
```
3. 创建数据访问接口
我们需要定义一个数据访问接口LeaveApplicationRepository,用来操作请假申请表。代码如下:
```java
import org.springframework.data.jpa.repository.JpaRepository;
public interface LeaveApplicationRepository extends JpaRepository<LeaveApplication, Integer> {
}
```
这里我们使用了Spring Data JPA框架,只需要继承JpaRepository接口即可。
4. 创建业务逻辑接口和实现类
我们需要定义一个业务逻辑接口LeaveApplicationService来处理请假申请的相关业务逻辑,代码如下:
```java
public interface LeaveApplicationService {
void addLeaveApplication(LeaveApplication leaveApplication);
void approveLeaveApplication(Integer id, Integer status);
List<LeaveApplication> getAllLeaveApplications();
}
```
其中,addLeaveApplication方法用来添加请假申请,approveLeaveApplication方法用来审批请假申请,getAllLeaveApplications方法用来获取所有请假申请的列表。
接着我们定义一个LeaveApplicationServiceImpl类来实现该接口,代码如下:
```java
@Service
public class LeaveApplicationServiceImpl implements LeaveApplicationService {
@Autowired
private LeaveApplicationRepository leaveApplicationRepository;
@Override
public void addLeaveApplication(LeaveApplication leaveApplication) {
leaveApplicationRepository.save(leaveApplication);
}
@Override
public void approveLeaveApplication(Integer id, Integer status) {
LeaveApplication leaveApplication = leaveApplicationRepository.getOne(id);
leaveApplication.setStatus(status);
leaveApplicationRepository.save(leaveApplication);
}
@Override
public List<LeaveApplication> getAllLeaveApplications() {
return leaveApplicationRepository.findAll();
}
}
```
这里我们使用了Spring框架的注解@Service来标记LeaveApplicationServiceImpl类为一个服务类,使用@Autowired注解自动注入LeaveApplicationRepository对象。
5. 创建前端页面
我们可以使用Thymeleaf模板引擎来创建前端页面,代码如下:
```html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>请假申请</title>
</head>
<body>
<h1>请假申请</h1>
<form th:action="@{/leave}" th:object="${leaveApplication}" method="post">
<div>
<label>姓名:</label>
<input type="text" th:field="*{name}" required>
</div>
<div>
<label>开始时间:</label>
<input type="datetime-local" th:field="*{startTime}" required>
</div>
<div>
<label>结束时间:</label>
<input type="datetime-local" th:field="*{endTime}" required>
</div>
<div>
<label>请假原因:</label>
<textarea th:field="*{reason}" required></textarea>
</div>
<button type="submit">提交申请</button>
</form>
<h1>请假申请列表</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>姓名</th>
<th>开始时间</th>
<th>结束时间</th>
<th>请假原因</th>
<th>审批状态</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr th:each="leaveApplication : ${leaveApplications}">
<td th:text="${leaveApplication.id}"></td>
<td th:text="${leaveApplication.name}"></td>
<td th:text="${#temporals.format(leaveApplication.startTime, 'yyyy-MM-dd HH:mm:ss')}"></td>
<td th:text="${#temporals.format(leaveApplication.endTime, 'yyyy-MM-dd HH:mm:ss')}"></td>
<td th:text="${leaveApplication.reason}"></td>
<td>
<span th:if="${leaveApplication.status == 0}">未审批</span>
<span th:if="${leaveApplication.status == 1}">已通过</span>
<span th:if="${leaveApplication.status == 2}">未通过</span>
</td>
<td>
<button th:if="${leaveApplication.status == 0}" th:onclick="'javascript:approve(' + ${leaveApplication.id} + ', 1);'">通过</button>
<button th:if="${leaveApplication.status == 0}" th:onclick="'javascript:approve(' + ${leaveApplication.id} + ', 2);'">不通过</button>
</td>
</tr>
</tbody>
</table>
<script type="text/javascript">
function approve(id, status) {
if (confirm("确定要审批吗?")) {
var form = document.createElement("form");
form.action = "/leave/" + id + "/" + status;
form.method = "post";
document.body.appendChild(form);
form.submit();
}
}
</script>
</body>
</html>
```
这个页面包括了请假申请表单和请假申请列表两个部分,使用Thymeleaf模板引擎将Java对象转换为HTML页面。其中,form表单用来提交请假申请,table表格用来显示请假申请列表。在按钮的onclick事件中,调用JavaScript函数approve来提交审批结果。
6. 创建控制器类
最后我们需要定义一个控制器类LeaveApplicationController来处理前端页面的请求和响应,代码如下:
```java
@Controller
public class LeaveApplicationController {
@Autowired
private LeaveApplicationService leaveApplicationService;
@GetMapping("/")
public String index(Model model) {
model.addAttribute("leaveApplication", new LeaveApplication());
model.addAttribute("leaveApplications", leaveApplicationService.getAllLeaveApplications());
return "index";
}
@PostMapping("/leave")
public String addLeaveApplication(@ModelAttribute LeaveApplication leaveApplication) {
leaveApplicationService.addLeaveApplication(leaveApplication);
return "redirect:/";
}
@PostMapping("/leave/{id}/{status}")
public String approveLeaveApplication(@PathVariable Integer id, @PathVariable Integer status) {
leaveApplicationService.approveLeaveApplication(id, status);
return "redirect:/";
}
}
```
这里我们使用了Spring框架的注解@Controller来标记LeaveApplicationController类为一个控制器类,使用@Autowired注解自动注入LeaveApplicationService对象。
在index方法中,我们使用Model对象将LeaveApplication对象和所有LeaveApplication对象的列表添加到模板中,然后返回index页面。在addLeaveApplication方法中,我们调用LeaveApplicationService的addLeaveApplication方法来添加一条请假申请,并重定向到index页面。在approveLeaveApplication方法中,我们调用LeaveApplicationService的approveLeaveApplication方法来审批一条请假申请,并重定向到index页面。
这样就完成了一个简单的Java实现请假功能的示例代码。
相关推荐
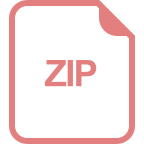
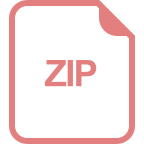
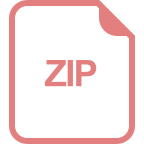














