document.currentScript 怎样转为Blob对象
时间: 2024-02-01 11:03:46 浏览: 145
`document.currentScript` 变量返回正在执行的 `<script>` 元素,可以用于获取当前脚本的 URL。如果你想要将当前脚本转换为 `Blob` 对象,可以使用以下方法:
1. 获取当前脚本的 URL
```javascript
const scriptUrl = document.currentScript.src;
```
2. 使用 `XMLHttpRequest` 对象获取脚本文件内容
```javascript
const xhr = new XMLHttpRequest();
xhr.open('GET', scriptUrl, true);
xhr.responseType = 'blob';
xhr.onload = () => {
const blob = xhr.response;
console.log(blob);
};
xhr.send();
```
在这个代码片段中,我们首先获取了当前脚本的 URL,然后使用 `XMLHttpRequest` 对象向服务器发送 GET 请求,获取脚本文件内容。设置 `responseType` 为 `blob`,表示响应类型为 `Blob` 对象。当服务器返回响应时,我们使用 `response` 属性获取响应内容,这里就是表示脚本文件内容的 `Blob` 对象。
需要注意的是,由于跨域安全限制,如果脚本文件不在同一个域名下,使用上述方法获取脚本文件内容可能会失败。此时,你可以考虑使用服务器端代理或者 CORS(跨域资源共享)等技术来解决跨域问题。
相关问题
export function convertRes2Blob(response,exportFileName) { // try { const fileName = response.headers['content-disposition']?.match(/filename=(.*)/)[1] ?? exportFileName // 将二进制流转为blob const blob = new Blob([response.data], { type: 'application/octet-stream' }) if (typeof window.navigator.msSaveBlob !== 'undefined') { // 兼容IE,window.navigator.msSaveBlob:以本地方式保存文件 window.navigator.msSaveBlob(blob, decodeURI(fileName)) } else { // 创建新的URL并指向File对象或者Blob对象的地址 const blobURL = window.URL.createObjectURL(blob) // 创建a标签,用于跳转至下载链接 const tempLink = document.createElement('a') tempLink.style.display = 'none' tempLink.href = blobURL tempLink.setAttribute('download', decodeURI(fileName)) // 兼容:某些浏览器不支持HTML5的download属性 if (typeof tempLink.download === 'undefined') { tempLink.setAttribute('target', '_blank') } // 挂载a标签 document.body.appendChild(tempLink) tempLink.click() document.body.removeChild(tempLink) // 释放blob URL地址 window.URL.revokeObjectURL(blobURL) } message.success("已导出"); // } // catch (error) { // message.warning("导出异常,请重新尝试"); // } } 导出报Uncaught (in promise) TypeError: Cannot read properties of undefined (reading 'content-disposition'是因为什么原因
这个错误通常是因为响应头中不存在 "content-disposition" 属性。在你的代码中,你尝试使用 response.headers['content-disposition'] 获取文件名,然而如果响应头中没有这个属性,则会返回 undefined,导致你在尝试使用 undefined.match(/filename=(.*)/)[1] 访问一个不存在的属性,从而导致这个错误。
为了避免这个问题,你可以在读取 response.headers['content-disposition'] 之前先检查一下这个属性是否存在,例如:
```
const fileName = (response.headers['content-disposition'] && response.headers['content-disposition'].match(/filename=(.*)/)[1]) || exportFileName;
```
这样,如果响应头中不存在 "content-disposition" 属性,fileName 将会默认使用传入的 exportFileName。
console.log(data); if (data.size > 0) { let blob = new Blob([data], { type: "application/pdf;charset-UTF-8", }); let fileName = title; if ("download" in document.createElement("a")) { const elink = document.createElement("a"); elink.download = fileName + type; elink.style.display = "none"; elink.href = URL.createObjectURL(blob); document.body.appendChild(elink); elink.click(); URL.revokeObjectURL(elink.href); document.body.removeChild(elink); } else { navigator.msSaveBlob(blob, fileName); } }
这段代码的作用是在浏览器中下载一个 PDF 文件。首先,它会将接收到的数据 `data` 打印到控制台。然后,它会检查数据的大小是否大于 0。如果是的话,就会创建一个包含数据的 Blob 对象,并设置其类型为 "application/pdf;charset-UTF-8"。接着,根据文件的标题 `title` 创建一个文件名 `fileName`。如果浏览器支持使用 `<a>` 标签的 `download` 属性下载文件,就会创建一个 `<a>` 元素,并设置其下载属性和链接。然后将该元素添加到 `document.body` 中,并模拟点击该元素进行下载,之后撤销链接并从 `document.body` 中移除该元素。如果浏览器不支持使用 `<a>` 标签下载文件,则会调用 `navigator.msSaveBlob` 方法进行下载。
阅读全文
相关推荐
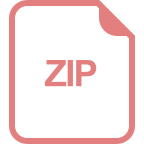
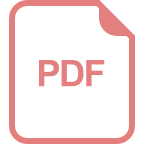

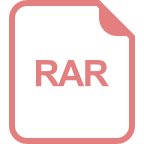
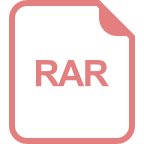
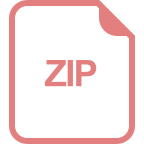
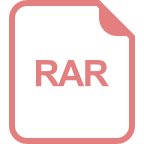
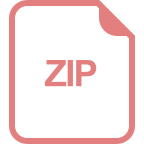
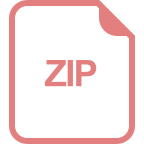






