python画太极八卦图
时间: 2023-06-04 07:06:37 浏览: 103
好的,让我来为您解答。要画出太极八卦图,您可以使用Python中的turtle模块来实现。具体步骤可参考以下代码:
```python
import turtle
# 设置窗口大小和背景颜色
turtle.setup(500, 500)
turtle.bgcolor("white")
# 设置画笔颜色和形状
turtle.color("black")
turtle.pensize(2)
# 画圆
turtle.penup()
turtle.goto(0, -150)
turtle.pendown()
turtle.circle(150)
# 画八卦
turtle.penup()
turtle.goto(0, -25)
turtle.pendown()
turtle.circle(125, 180)
turtle.circle(25, 180)
turtle.penup()
turtle.goto(0, -50)
turtle.pendown()
turtle.circle(-125, 180)
turtle.circle(-25, 180)
# 显示结果
turtle.hideturtle()
turtle.done()
```
希望能帮助到您。
相关问题
python画太极八卦图代码
以下是Python画太极八卦图的代码:
```python
import turtle as t
# 设置背景和笔的移动速度及大小
t.bgcolor('yellow')
t.speed(10)
t.pensize(6)
# 画黑色的半圆
t.fillcolor("black")
t.begin_fill()
t.circle(100,180)
t.left(0)
t.circle(50,180)
t.circle(-50,180)
t.end_fill()
# 画白色的半圆
t.fillcolor("white")
t.begin_fill()
t.circle(-100,180)
t.left(180)
t.circle(50,180)
t.circle(-50,180)
t.end_fill()
# 在黑圆画白色的点
t.left(180)
t.up()
t.goto(10,150)
t.down()
t.pencolor("white")
t.fillcolor("white")
t.begin_fill()
t.circle(13)
t.end_fill()
# 在白圆画黑色的点
t.up()
t.goto(-10,30)
t.fillcolor("black")
.begin_fill()
t.circle(15)
t.end_fill()
t.pencolor('blue')
t.goto(1000,1000)
t.done()
```
python画一个太极八卦图
以下是使用Python画太极八卦图的代码示例:
```python
import turtle
# 设置画布大小和背景颜色
turtle.setup(800, 600)
turtle.bgcolor("black")
# 设置画笔颜色和大小
turtle.pensize(3)
turtle.pencolor("white")
# 绘制太极图
turtle.speed(10)
# 绘制上半部分
turtle.fillcolor("black")
turtle.begin_fill()
turtle.circle(150, 180)
turtle.circle(75, 180)
turtle.left(180)
turtle.circle(-75, 180)
turtle.end_fill()
# 绘制下半部分
turtle.fillcolor("white")
turtle.begin_fill()
turtle.circle(-150, 180)
turtle.circle(-75, 180)
turtle.left(180)
turtle.circle(75, 180)
turtle.end_fill()
# 绘制两个小圆
turtle.penup()
turtle.goto(0, 150)
turtle.pendown()
turtle.fillcolor("black")
turtle.begin_fill()
turtle.circle(30)
turtle.end_fill()
turtle.penup()
turtle.goto(0, -150)
turtle.pendown()
turtle.fillcolor("white")
turtle.begin_fill()
turtle.circle(30)
turtle.end_fill()
# 隐藏画笔
turtle.hideturtle()
# 结束绘制
turtle.done()
```
相关推荐
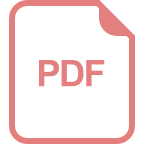








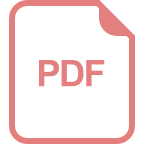
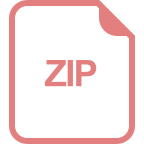
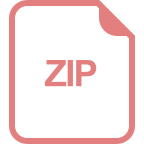
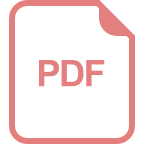