pygame怎么控制图片旋转
时间: 2023-10-29 11:47:05 浏览: 27
This error message is indicating that you are trying to access an element in a one-dimensional array that does not exist. Specifically, you are trying to access the element at index -9, which is outside the bounds of the array.
The size of the array is 1, which means that it only has one element. Therefore, the only valid index for this array is 0.
To fix this error, you need to make sure that you are using valid indices when accessing elements in the array.
相关问题
pygame怎么控制图片展示角度
要控制图片展示角度,可以使用pygame中的transform模块中的函数,例如:
1. rotate:旋转图像
```
rotated_image = pygame.transform.rotate(image, angle)
```
其中,image为原始图像,angle为要旋转的角度。
2. flip:翻转图像
```
flipped_image = pygame.transform.flip(image, xbool, ybool)
```
其中,image为原始图像,xbool和ybool分别表示是否在x轴和y轴上进行翻转。
3. scale:缩放图像
```
scaled_image = pygame.transform.scale(image, (width, height))
```
其中,image为原始图像,width和height分别表示缩放后的宽度和高度。
使用这些函数可以对图片进行各种角度的控制和变换。
怎么用pygame让图片绕定点旋转
### 回答1:
要用 Pygame 实现图片的旋转,你可以使用 Pygame 中的 `transform` 模块中的 `rotate()` 函数。
下面是一个使用 Pygame 旋转图像的简单示例:
```
import pygame
pygame.init()
# 加载图像
image = pygame.image.load('image.png')
# 获取图像中心点
image_center = image.get_rect().center
# 创建 Pygame 窗口
screen = pygame.display.set_mode((640, 480))
# 旋转角度
angle = 45
# 旋转图像
rotated_image = pygame.transform.rotate(image, angle)
# 将旋转后的图像绘制到屏幕上
screen.blit(rotated_image, (320, 240))
# 更新屏幕显示
pygame.display.flip()
# 等待窗口关闭事件
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
```
在上面的代码中,`rotate()` 函数接收两个参数:要旋转的图像和旋转的角度。为了将图像旋转到指定的角度,我们需要使用 `get_rect()` 方法获取图像的矩形,并使用 `center` 属性获取图像的中心点。我们然后创建一个 Pygame 窗口,使用 `rotate()` 函数旋转图像,并使用 `blit()` 函数将旋转后的图像绘制到屏幕上。最后,我们更新屏幕显示并等待窗口关闭事件。
### 回答2:
在使用pygame让图片绕定点旋转时,可以按照以下步骤进行操作:
1. 导入必要的模块和库:
```
import pygame
import math
```
2. 初始化pygame:
```
pygame.init()
```
3. 创建游戏窗口和画布:
```
window_width = 800
window_height = 600
screen = pygame.display.set_mode((window_width, window_height))
canvas = pygame.Surface(screen.get_size())
```
4. 加载图片并设置旋转中心点:
```
image = pygame.image.load("path_to_image.png") # 替换成实际的图片路径
image_rect = image.get_rect()
rotation_center = image_rect.center # 设置旋转中心为图片的中心点
```
5. 设置旋转角度:
```
angle = 0 # 初始旋转角度为0度
rotation_speed = 1 # 旋转速度(可根据实际需求调整)
```
6. 游戏主循环:
```
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 控制旋转角度
angle += rotation_speed
if angle >= 360:
angle = 0
# 清除画布
canvas.fill((0, 0, 0))
# 在画布上绘制旋转后的图片
rotated_image = pygame.transform.rotate(image, angle)
rotated_rect = rotated_image.get_rect(center=rotation_center)
canvas.blit(rotated_image, rotated_rect)
# 刷新屏幕
screen.blit(canvas, (0, 0))
pygame.display.flip()
```
7. 退出游戏:
```
pygame.quit()
```
以上就是使用pygame让图片绕定点旋转的简单实现。在游戏主循环中,我们通过不断更新旋转角度和在画布上绘制旋转后的图片来实现图像的旋转效果。可以根据实际需求调整旋转的速度和旋转中心点。
### 回答3:
要使用Pygame让图片绕固定点旋转,我们需要使用Pygame中的transform模块和角度计算函数。
首先,我们需要加载图片并指定旋转的角度。可以使用Pygame中的`pygame.image.load()`函数加载图片,然后使用`pygame.transform.rotate()`函数选择角度进行旋转。
接下来,我们需要指定旋转的中心点。在Pygame中,图片旋转的中心点是图片的矩形的中心点。可以使用`get_rect()`函数获取图片矩形的位置和大小,然后使用`centerx`和`centery`属性获取中心点的x和y坐标。
然后,我们需要使用`blit()`函数在游戏窗口中绘制旋转后的图片。可以使用`pygame.display.set_mode()`函数创建一个窗口,再使用`blit()`函数将旋转后的图片绘制到窗口中。
最后,通过调用`pygame.display.flip()`函数来更新窗口,以显示旋转后的图片。
下面是一个示例代码:
```python
import pygame
pygame.init()
# 创建窗口
window_width = 800
window_height = 600
window = pygame.display.set_mode((window_width, window_height))
# 加载图片
image = pygame.image.load("image.png")
image_rect = image.get_rect()
# 设置旋转角度
angle = 45
# 计算旋转后的中心点
center_x = image_rect.centerx
center_y = image_rect.centery
# 旋转图片
rotated_image = pygame.transform.rotate(image, angle)
# 将旋转后的图片绘制到窗口中心
rotated_rect = rotated_image.get_rect(center=(center_x, center_y))
window.blit(rotated_image, rotated_rect)
# 更新窗口
pygame.display.flip()
# 游戏循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
pygame.quit()
```
这样,我们就可以使用Pygame让图片绕固定点旋转了。以上示例代码中,我们将图片旋转了45度,并绘制在窗口中心。可以根据自己的需求修改旋转的角度和绘制的位置。
相关推荐
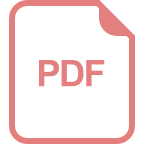
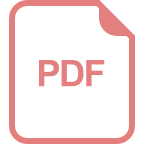








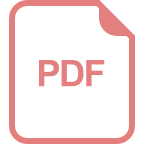
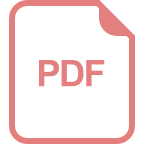