基于HK32F030MF4P6配置GPIO来控制TM1650芯片进而控制数码管,要求写出配置函数和驱动函数
时间: 2024-04-08 17:34:15 浏览: 18
好的,以下是一个示例的配置函数和驱动函数,用于基于HK32F030MF4P6配置GPIO来控制TM1650芯片进而控制数码管:
```c
#include "stm32f0xx.h"
#define TM1650_SCL_PIN GPIO_Pin_10
#define TM1650_SDA_PIN GPIO_Pin_11
#define TM1650_GPIO_PORT GPIOB
void TM1650_GPIO_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_AHBPeriphClockCmd(RCC_AHBPeriph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = TM1650_SCL_PIN | TM1650_SDA_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_OType = GPIO_OType_OD;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(TM1650_GPIO_PORT, &GPIO_InitStructure);
}
void TM1650_Start(void)
{
GPIO_SetBits(TM1650_GPIO_PORT, TM1650_SCL_PIN);
GPIO_SetBits(TM1650_GPIO_PORT, TM1650_SDA_PIN);
GPIO_ResetBits(TM1650_GPIO_PORT, TM1650_SDA_PIN);
GPIO_ResetBits(TM1650_GPIO_PORT, TM1650_SCL_PIN);
}
void TM1650_Stop(void)
{
GPIO_ResetBits(TM1650_GPIO_PORT, TM1650_SCL_PIN);
GPIO_ResetBits(TM1650_GPIO_PORT, TM1650_SDA_PIN);
GPIO_SetBits(TM1650_GPIO_PORT, TM1650_SCL_PIN);
GPIO_SetBits(TM1650_GPIO_PORT, TM1650_SDA_PIN);
}
void TM1650_WriteByte(uint8_t data)
{
uint8_t i;
for (i = 0; i < 8; i++)
{
GPIO_ResetBits(TM1650_GPIO_PORT, TM1650_SCL_PIN);
if (data & 0x80)
GPIO_SetBits(TM1650_GPIO_PORT, TM1650_SDA_PIN);
else
GPIO_ResetBits(TM1650_GPIO_PORT, TM1650_SDA_PIN);
GPIO_SetBits(TM1650_GPIO_PORT, TM1650_SCL_PIN);
data <<= 1;
}
GPIO_ResetBits(TM1650_GPIO_PORT, TM1650_SCL_PIN);
GPIO_SetBits(TM1650_GPIO_PORT, TM1650_SDA_PIN);
GPIO_SetBits(TM1650_GPIO_PORT, TM1650_SCL_PIN);
GPIO_ResetBits(TM1650_GPIO_PORT, TM1650_SCL_PIN);
}
void TM1650_DisplayNumber(uint8_t num)
{
uint8_t displayData = numToTM1650Data(num);
TM1650_Start();
TM1650_WriteByte(0x44); // Command to set display brightness
TM1650_Stop();
TM1650_Start();
TM1650_WriteByte(0x40); // Command to set data
TM1650_WriteByte(displayData); // Send data to display
TM1650_Stop();
}
uint8_t numToTM1650Data(uint8_t num)
{
static const uint8_t segmentData[] = {
0x3F, // 0
0x06, // 1
0x5B, // 2
0x4F, // 3
0x66, // 4
0x6D, // 5
0x7D, // 6
0x07, // 7
0x7F, // 8
0x6F // 9
};
if (num > 9)
num = 0; // Display '0' for numbers out of range
return segmentData[num];
}
```
在上述示例中,我们使用了STM32F0系列的库函数来配置GPIO。首先,我们使能了GPIOB的时钟。然后,我们定义了TM1650_SCL_PIN和TM1650_SDA_PIN分别对应TM1650的SCL和SDA引脚。接下来,我们使用GPIO_InitTypeDef结构体来配置GPIO引脚的模式、速度、输出类型和上下拉电阻。最后,我们调用GPIO_Init函数来初始化GPIO引脚。
在驱动函数中,我们实现了TM1650通信的基本操作函数。TM1650_Start函数发送起始信号,TM1650_Stop函数发送停止信号。TM1650_WriteByte函数用于发送一个字节的数据到TM1650。TM1650_DisplayNumber函数用于将数字显示在数码管上,通过调用TM1650_Start、TM1650_WriteByte等函数来发送相应的命令和数据到TM1650。
最后,numToTM1650Data函数用于将数字转换为TM1650可以识别的数据格式。在该函数中,我们定义了一个静态数组segmentData,通过索引来获取对应数字的数据。
请根据您的实际需求修改上述代码,并根据您的实际情况进行连接和使用。希望对您有所帮助!如有任何疑问,请随时向我提问。
相关推荐
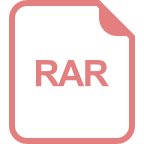
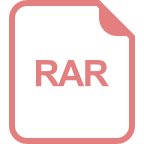














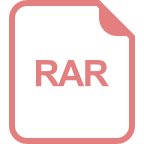