<?xml version="1.0" encoding="ISO-8859-1"?> <bookstore> <book category="children"> <author>J K. Rowling</author> <year>2005</year> <price>29.99</price> </book> <book category="cooking"> <author>Giada De Laurentiis</author> <year>2005</year> <price>30.00</price> </book> <book category="web"> <author>Erik T. Ray</author> <year>2003</year> <price>39.95</price> </book> <book category="web"> <author>James McGovern</author> <author>Per Bothner</author> <author>Kurt Cagle</author> <author>James Linn</author> <author>Vaidyanathan Nagarajan</author> <year>2003</year> <price>49.99</price> </book> </bookstore> 按照上述xml文件,使用html和javascript读取.xml文件的节点内容,以表格方式对内容进行显示,通过下拉单进行交互式书目内容选取,仅显示选中的书目信息
时间: 2023-07-02 13:05:45 浏览: 182
以下是实现该功能的示例代码:
HTML部分:
```html
<!DOCTYPE html>
<html>
<head>
<title>Interactive Bookstore</title>
</head>
<body>
<select id="category">
<option value="">Select a category</option>
<option value="children">Children</option>
<option value="cooking">Cooking</option>
<option value="web">Web</option>
</select>
<table id="bookTable">
<thead>
<tr>
<th>Author</th>
<th>Year</th>
<th>Price</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
<script src="script.js"></script>
</body>
</html>
```
JavaScript部分:
```javascript
// 加载xml文件
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
displayBooks(this);
}
};
xhttp.open("GET", "books.xml", true);
xhttp.send();
// 处理选择器变化事件
document.getElementById("category").addEventListener("change", function() {
var selectedCategory = this.value;
var books = document.getElementsByTagName("book");
// 遍历所有书籍,根据类别显示或隐藏
for (var i = 0; i < books.length; i++) {
if (selectedCategory == "" || books[i].getAttribute("category") == selectedCategory) {
books[i].style.display = "table-row";
} else {
books[i].style.display = "none";
}
}
});
// 显示所有书籍
function displayBooks(xml) {
var xmlDoc = xml.responseXML;
var books = xmlDoc.getElementsByTagName("book");
var bookTable = document.getElementById("bookTable");
// 遍历所有书籍,并在表格中添加新行
for (var i = 0; i < books.length; i++) {
var row = bookTable.insertRow(-1);
row.setAttribute("category", books[i].getAttribute("category"));
row.style.display = "table-row";
var authorCell = row.insertCell(0);
var yearCell = row.insertCell(1);
var priceCell = row.insertCell(2);
authorCell.innerHTML = books[i].getElementsByTagName("author")[0].childNodes[0].nodeValue;
yearCell.innerHTML = books[i].getElementsByTagName("year")[0].childNodes[0].nodeValue;
priceCell.innerHTML = books[i].getElementsByTagName("price")[0].childNodes[0].nodeValue;
}
}
```
在该示例中,我们首先使用XMLHttpRequest对象加载books.xml文件。一旦文件加载完成,我们调用displayBooks函数来解析XML并在表格中显示所有书籍信息。
我们还在页面上添加了一个下拉单,使用户可以选择要显示的书籍类别。在选择器变化事件中,我们遍历所有书籍,并根据类别显示或隐藏它们。
在表格中显示书籍信息时,我们使用insertRow函数向表格添加新行,并使用insertCell函数向行添加新单元格。我们还使用getAttribute和getElementsByTagName函数从XML中获取书籍的类别、作者、年份和价格信息。
阅读全文
相关推荐
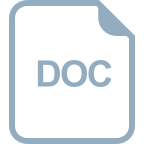
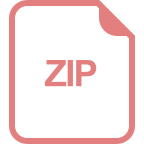
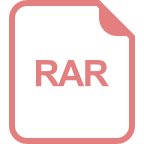


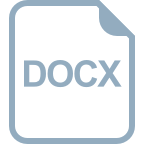
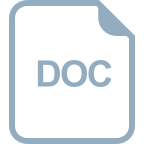
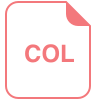
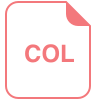
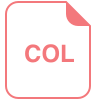
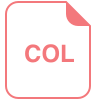
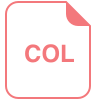
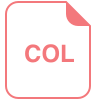




