java编程一个程序,4个线程同时向一个10000位的数组中分别写入1-10,100-1000,2000-3000,4000-5000的数。
时间: 2024-02-20 18:56:09 浏览: 28
下面是一个基于Java的实现代码,使用了四个线程并行写入指定范围内的数字到数组中:
```
public class Main {
public static void main(String[] args) {
int[] arr = new int[10000];
Thread t1 = new Thread(new MyThread(arr, 0, 10));
Thread t2 = new Thread(new MyThread(arr, 100, 1000));
Thread t3 = new Thread(new MyThread(arr, 2000, 3000));
Thread t4 = new Thread(new MyThread(arr, 4000, 5000));
t1.start();
t2.start();
t3.start();
t4.start();
try {
t1.join();
t2.join();
t3.join();
t4.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Arrays.toString(arr));
}
}
class MyThread implements Runnable {
private int[] arr;
private int start;
private int end;
public MyThread(int[] arr, int start, int end) {
this.arr = arr;
this.start = start;
this.end = end;
}
@Override
public void run() {
for (int i = start; i <= end; i++) {
arr[i] = i;
}
}
}
```
在主函数中,我们先创建一个长度为10000的整型数组。然后创建四个线程,并将它们的任务分别指定为写入1-10、100-1000、2000-3000、4000-5000的数。接着启动这四个线程,等待它们全部执行完毕后,打印输出数组的内容。
在每个线程的任务中,我们使用一个简单的循环来将指定范围内的数字写入数组中。由于四个线程是并行执行的,所以它们会同时写入不同的位置,因此不需要考虑线程安全的问题。最后,主函数中输出数组的内容,我们可以看到数组中已经成功地写入了指定范围内的数字。
相关推荐
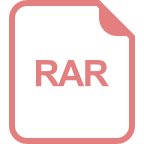
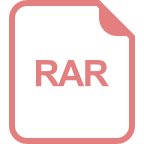
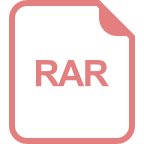














