用c语言写出 以下是C语言的代码```c #include <stdio.h#include <ctype.h> // 头ctype.h中包含tolower函数 main() { char string[]; // 定义一个字符数组,于存储用户输入的字符串 printf("请输入一个字符串: scanf("%s", string); 从键盘输入一个字符串,储到string数组中 for (int i = 0 string[i] != '\0 i++) { // 遍历字符串中每一个字符 ifisupper(string[i])) { //字符是否为大写字 string[i] = to(string[i]); // 将大写字转换成小写字 } } printf("转换后的为:%s", string); // 输出换后的字符串 return ; } ``` 该代码中了头文件ctype.h中的两函数:isupper和tolower。is函数用于判断字符是否大写字母,而函数用于将大写母转换成小写字。首先通过scanf函数从盘获取用户输入的字符串,历字符串中的每一个,若为大写字母则转换成小字母后输出的代码
时间: 2023-12-14 17:36:05 浏览: 70
如下:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char string[100]; // 定义一个字符数组,用于存储用户输入的字符串
printf("请输入一个字符串:");
scanf("%s", string); // 从键盘输入一个字符串,存储到string数组中
for (int i = 0; string[i] != '\0'; i++) { // 遍历字符串中每一个字符
if (isupper(string[i])) { // 字符是否为大写字母
string[i] = tolower(string[i]); // 将大写字母转换成小写字母
}
}
printf("转换后的为:%s\n", string); // 输出转换后的字符串
return 0;
}
```
需要注意的是,代码中的字符数组大小应该足够大,以储存用户可能输入的任意长度字符串。此外,程序最后需要返回0作为正常结束的标志。
相关问题
#include <stdio.h> #include <stdlib.h> #include <stdint.h> #include <string.h> #include <unistd.h> #include <fcntl.h> #include <pthread.h> #include <sys/types.h> #include <sys/socket.h> #include <netinet/in.h> #include <ctype.h> #include <sys/pps.h> #include <sys/types.h> #include "camera.h" #include <encoder.h> #include "ppsFunc.h" #include "timeDiff.h"
这是一段C语言的代码,使用了一些系统库和自定义头文件。其中包括了网络编程相关的库,如sys/socket.h和netinet/in.h,以及多线程相关的库pthread.h。另外还有一些自定义的头文件,如camera.h和encoder.h,可能是该程序所需的一些外部依赖。该程序可能是一个视频流的采集和编码程序,同时还使用了PPS和时间差等功能。
#include <ctype.h>
C语言的头文件`<ctype.h>`提供了一些用于测试和映射字符的函数。这些函数可以用于判断字符是否为字母、数字或空格,以及将字符转换为大写或小写。以下是一些常用的函数:
1. `isalpha(int c)`:判断字符是否为字母。
2. `isdigit(int c)`:判断字符是否为数字。
3. `isspace(int c)`:判断字符是否为空格。
4. `isupper(int c)`:判断字符是否为大写字母。
5. `islower(int c)`:判断字符是否为小写字母。
6. `toupper(int c)`:将字符转换为大写字母。
7. `tolower(int c)`:将字符转换为小写字母。
这些函数的参数是一个整数,通常是一个字符的ASCII码。如果参数所表示的字符具有相应的属性,则函数返回非零值,否则返回零。
例如,下面的代码演示了如何使用`<ctype.h>`头文件中的函数来判断一个字符是否为字母或数字:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char c = 'A';
if (isalpha(c)) {
printf("%c is an alphabet.\n", c);
} else {
printf("%c is not an alphabet.\n", c);
}
c = '5';
if (isdigit(c)) {
printf("%c is a digit.\n", c);
} else {
printf("%c is not a digit.\n", c);
}
return 0;
}
```
输出结果为:
```
A is an alphabet.
5 is a digit.
```
阅读全文
相关推荐
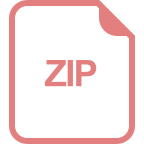
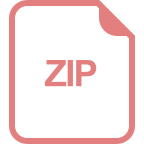
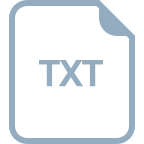













