在a.js用XMLHttpRequest 封装一个HttpGet请求函数,在a.html 引用这个函数发送请求,并在a.html打印返回值,直接给出代码示例
时间: 2023-08-31 14:32:21 浏览: 126
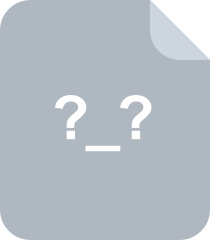
JS中基于XMLHttpRequest的Get 请求demo
### 回答1:
a.js:function httpGet(url) {
var xhr = new XMLHttpRequest();
xhr.open("GET", url, true);
xhr.send();
xhr.onreadystatechange = function () {
if (xhr.readyState == 4) {
if (xhr.status == 200) {
return xhr.responseText;
}
}
}
}a.html:<script type="text/javascript" src="a.js"></script>
<script type="text/javascript">
var res = httpGet("your url");
console.log(res);
</script>
### 回答2:
在a.js中封装一个HttpGet请求函数,代码如下:
```javascript
function httpGet(url, callback) {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
callback(xhr.responseText);
}
};
xhr.open("GET", url, true);
xhr.send();
}
```
在a.html中引用这个函数发送请求,并在a.html页面打印返回值,代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>a.html</title>
<script src="a.js"></script>
</head>
<body>
<script>
httpGet("http://example.com/api", function(responseText) {
console.log(responseText);
});
</script>
</body>
</html>
```
在a.html中引用a.js文件,并在页面加载完成后调用httpGet函数发送GET请求至"http://example.com/api"接口。当请求成功返回时,将返回值通过回调函数传递给console.log函数进行打印输出。
### 回答3:
a.js代码示例:
```javascript
function httpGet(url, callback) {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
callback(xhr.responseText);
}
};
xhr.open('GET', url, true);
xhr.send();
}
```
a.html代码示例:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>HttpGet请求示例</title>
<script src="a.js"></script>
<script>
function printResponse(response) {
console.log(response);
}
window.onload = function() {
httpGet('http://example.com/api/data', printResponse);
};
</script>
</head>
<body>
</body>
</html>
```
在a.html页面中,我们通过`<script src="a.js"></script>`引用了a.js文件中的httpGet函数。然后,在window.onload事件中调用httpGet函数发送GET请求,并传入一个回调函数printResponse来处理返回的结果。printResponse函数会将返回的结果打印到控制台中。
阅读全文
相关推荐







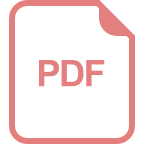
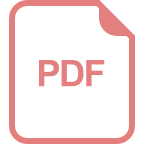
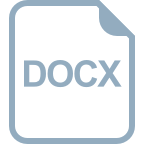
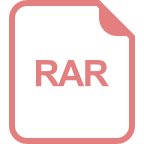
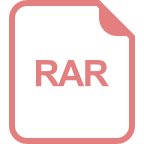
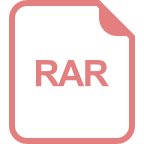
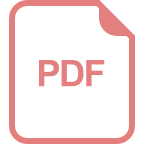
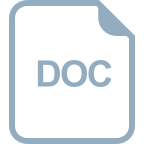
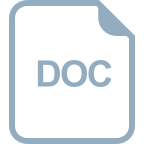