你现在是一个前端架构师,请你站在前端架构师的角度请基于Vue.js和Axios库写出一个文件分片上传的组件
时间: 2023-06-03 12:07:21 浏览: 129
好的,作为一个前端架构师,我可以基于Vue.js和Axios库写出一个文件分片上传的组件。
首先,我们需要引入Vue.js和Axios库。然后,我们可以创建一个Vue组件,命名为FileUploader。
接着,在组件的template中,我们可以添加一个文件选择器,让用户可以选择要上传的文件。同时,我们也可以添加一个上传按钮,让用户点击后可以开始上传文件。
然后,在组件的script中,我们可以定义一些状态变量,例如上传进度、分片数量等等。
下一步,我们可以使用Axios库来实现文件分片上传。具体操作是,将文件分成若干个固定大小的分片,然后依次上传这些分片。在上传过程中,我们可以实时更新上传进度,让用户看到当前上传的进度。
最后,在组件的样式中,我们可以定义上传进度条样式、按钮样式等等,让组件更加美观和易用。
下面是一个示例代码,供您参考:
```
<template>
<div>
<input type="file" @change="onFileSelected" />
<button @click="uploadFile">上传</button>
<div class="progress">
<div class="progress-bar" :style="{ width: progress + '%' }"></div>
</div>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
file: null,
chunks: [],
progress: 0
};
},
methods: {
onFileSelected(event) {
this.file = event.target.files[0];
},
async uploadFile() {
const chunkSize = 1024 * 1024; // 1MB
const totalSize = this.file.size;
const chunkCount = Math.ceil(totalSize / chunkSize);
let currentChunk = 0;
while (currentChunk < chunkCount) {
const start = currentChunk * chunkSize;
const end = Math.min(start + chunkSize, totalSize);
const chunk = this.file.slice(start, end);
this.chunks.push(chunk);
currentChunk++;
}
this.progress = 0;
const config = {
onUploadProgress: progressEvent => {
this.progress = Math.round((progressEvent.loaded * 100) / progressEvent.total);
}
};
const baseUrl = 'http://your-api-url.com';
for (let i = 0; i < this.chunks.length; i++) {
const formData = new FormData();
formData.append('file', this.chunks[i], `${i}-${this.file.name}`);
formData.append('totalChunks', this.chunks.length);
formData.append('chunkIndex', i);
await axios.post(`${baseUrl}/upload`, formData, config);
}
alert('上传成功!');
}
}
};
</script>
<style>
.progress {
width: 100%;
height: 20px;
background-color: #cccccc;
margin-top: 10px;
}
.progress-bar {
height: 100%;
background-color: #007bff;
}
</style>
```
阅读全文
相关推荐
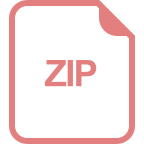
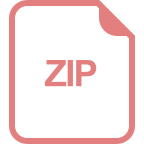

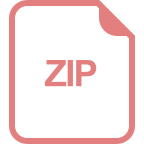
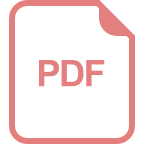
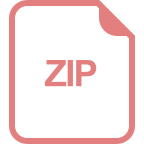
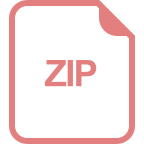
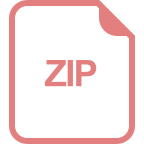
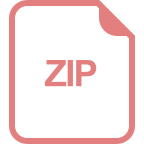
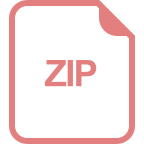
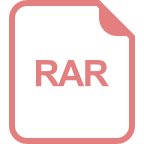
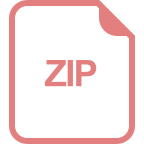
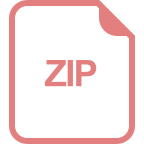
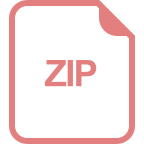
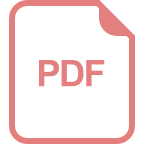
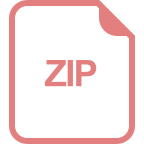
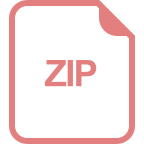
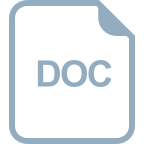