用java通过键盘输入模拟用户交流,然后把交流的信息记录在log.txt中。 --格式:【用户1】【时间yyyy-MM-dd HH:mm:ss】【内容】 --当其中用户一个输入exit时,聊天结束。
时间: 2024-02-17 12:04:27 浏览: 109
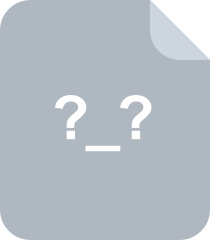
可模拟键盘上任何键,模拟输入,内带各种常用函数,有注释
好的,以下是Java的实现代码:
```java
import java.io.FileWriter;
import java.io.IOException;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import java.util.Scanner;
public class Main {
public static void main(String[] args) throws IOException {
String user1 = "用户1";
Scanner scanner = new Scanner(System.in);
FileWriter fileWriter = new FileWriter("log.txt");
while (true) {
System.out.print("请输入要发送的消息:");
String msg = scanner.nextLine();
if (msg.equals("exit")) {
break;
}
String logStr = "【" + user1 + "】【" + getCurrentTime() + "】【" + msg + "】";
System.out.println(logStr);
fileWriter.write(logStr + "\n");
fileWriter.flush();
}
fileWriter.close();
System.out.println("聊天已结束!");
}
// 获取当前时间
private static String getCurrentTime() {
LocalDateTime now = LocalDateTime.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
return now.format(formatter);
}
}
```
上述程序中,我使用了Java的`Scanner`类来实现从键盘输入消息的功能。在主程序中,我使用了一个无限循环来不断接收用户输入的消息。每次接收到消息后,程序会将其格式化成日志字符串,然后打印到控制台并写入到日志文件中。当用户输入"exit"时,程序会结束循环并输出提示信息。在程序中,我定义了一个`getCurrentTime()`方法,用于获取当前时间,并使用Java 8新增的日期时间API来格式化时间字符串。此外,我还使用了Java的`FileWriter`类来向日志文件中写入日志字符串。注意,在写入日志字符串后,需要调用`flush()`方法将缓冲区的内容刷新到磁盘中。最后,在程序结束前需要关闭`FileWriter`对象。
阅读全文
相关推荐
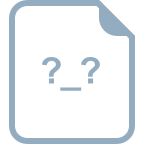
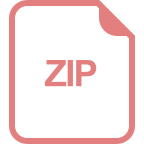
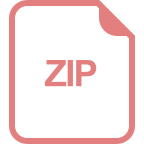
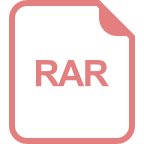
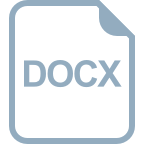
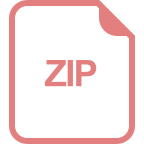
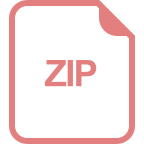
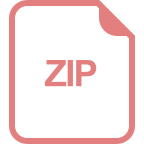
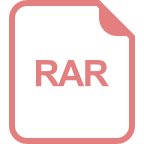
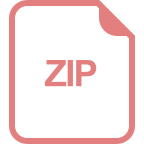
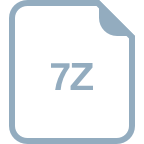
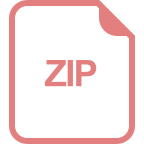
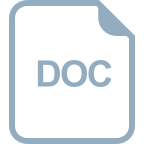
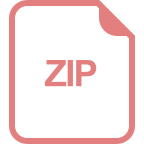
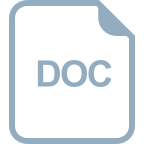
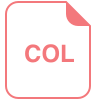
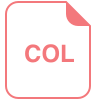