解释代码: def convert_to_pseudocolor(self): # 转换为8位伪彩色 palette = [(i, i, i) for i in range(256)] new_pixels = [] for row in self.pixels: new_row = [] for pixel in row: new_row.append(pixe
时间: 2024-02-10 17:22:27 浏览: 77
该代码是一个类的方法,用于将图像转换为8位伪彩色。具体解释如下:
1. 首先定义了一个颜色调色板palette,其中包含了256种灰度颜色,每种颜色的RGB值相等。
2. 然后定义了一个新的像素列表new_pixels用于存储新的伪彩色像素。
3. 通过循环遍历原图像素列表self.pixels,对于每一行像素,再次进行循环遍历,对于每个像素,将其灰度值作为索引值,从颜色调色板palette中取出对应的RGB值,作为新像素的颜色值。
4. 然后将新像素加入到新像素列表new_row中,最后将new_row加入到新像素列表new_pixels中。
5. 最终返回新像素列表new_pixels,完成了将原图像转换为伪彩色图像的操作。
相关问题
python读取一个 24 位真彩色 BMP 文件,然后转化为灰色图像,最后存储为 8 位伪彩色 BMP 文 件;
可以使用PIL库来处理这个问题,具体代码可以参考下面这段:
``` python
from PIL import Image
# 读取24位真彩色BMP文件
img = Image.open('input.bmp')
# 转化为灰度图像
img_gray = img.convert('L')
# 转化为8位伪彩色BMP文件
img_pseudo = img_gray.convert('P', palette=Image.ADAPTIVE, colors=256)
# 保存为伪彩色BMP文件
img_pseudo.save('output.bmp')
```
这段代码将输入的24位真彩色BMP文件转化为灰度图像,再将灰度图像转化为8位伪彩色BMP文件,并保存输出。
编写 Python 程序,读取D盘的一个 24 位真彩色 BMP 文件(地址:D:\多媒体技术\3\实验三素材\实验三素材\24位真彩色BMP),文件名为:robert.bmp,然后转化为灰色图像,最后存储为 8 位伪彩色 BMP 文件;要求使用面向对象的方式来写(使用 class),不能使用 Python 图像模块/包/库,如 opencv、PIL 等。在定义 class 里的成员时,可使用 int.to_bytes、int.from_bytes等。要详细一点的,包括读取文件。
我明白了,这是一道编程题,你想让我帮你编写一个可以将24位真彩色BMP文件转换为灰色图像,最后存储为8位伪彩色BMP文件的Python程序,对吧?
好的,先说一下步骤,我们可以将24位真彩色BMP文件的RGB值转换为灰度值,再将灰度值转换为8位伪彩色数值,最后输出8位伪彩色BMP文件,这个过程可以使用面向对象的方式来实现。
以下是代码示例:
```python
class BMPConverter:
def __init__(self, filepath):
self.filepath = filepath
def read_file(self):
with open(self.filepath, 'rb') as file:
# 读取文件头部,19-22位为位图数据偏移量
file.seek(18)
self.img_offset = int.from_bytes(file.read(4), byteorder='little')
# 读取位图宽度和高度,18-21位为宽度,22-25位为高度
file.seek(18)
self.width = int.from_bytes(file.read(4), byteorder='little')
self.height = int.from_bytes(file.read(4), byteorder='little')
# 读取位图数据
file.seek(self.img_offset)
self.data = file.read()
def to_gray(self):
# 将RGB值转换为灰度值
gray_data = bytearray()
for i in range(self.width * self.height):
b = self.data[i * 3]
g = self.data[i * 3 + 1]
r = self.data[i * 3 + 2]
gray_value = int(0.3 * r + 0.59 * g + 0.11 * b)
gray_data.append(gray_value)
self.data = gray_data
def to_pseudocolor(self):
# 将灰度值转换为8位伪彩色数值
pseudo_data = bytearray()
for i in range(self.width * self.height):
gray_value = self.data[i]
pseudo_value = int(gray_value / 16) # 每个伪彩色值跨度为16
pseudo_data.append(pseudo_value)
self.data = pseudo_data
def write_file(self, outpath):
# 输出8位伪彩色BMP文件
with open(outpath, 'wb') as file:
# 写入文件头部
file.write(b'BM')
file.write((14 + 40 + self.width * self.height).to_bytes(4, byteorder='little'))
file.write(b'\x00\x00')
file.write(b'\x00\x00')
file.write((14 + 40).to_bytes(4, byteorder='little'))
# 写入信息头
file.write(b'\x28\x00\x00\x00') # BITMAPINFOHEADER
file.write(self.width.to_bytes(4, byteorder='little'))
file.write(self.height.to_bytes(4, byteorder='little'))
file.write(b'\x01\x00') # 1个平面
file.write(b'\x08\x00') # 8位伪彩色
file.write(b'\x00\x00\x00\x00') # 不压缩
file.write((self.width * self.height).to_bytes(4, byteorder='little'))
file.write(b'\x00\x00\x00\x00')
file.write(b'\x00\x00\x00\x00')
file.write(b'\x00\x00\x00\x00')
file.write(b'\x00\x00\x00\x00')
# 写入调色板
for i in range(256):
file.write(bytes([i, i, i, 0]))
# 写入位图数据
file.write(self.data)
# 测试
if __name__ == '__main__':
converter = BMPConverter(r'D:\多媒体技术\3\实验三素材\实验三素材\24位真彩色BMP\robert.bmp')
converter.read_file()
converter.to_gray()
converter.to_pseudocolor()
converter.write_file('output.bmp')
```
这段程序会读取文件,将RGB值转换为灰度值,再将灰度值转换为8位伪彩色数值,最后输出8位伪彩色BMP文件,你可以根据需要修改代码中的输入输出路径。
阅读全文
相关推荐









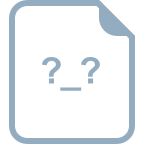





