在mongoDB中构建一个 Web 页面,以表格形式显示已结账的订单信息中,每个商品的名称和订单总额;(10 分)
时间: 2024-04-08 20:31:10 浏览: 98
要在MongoDB中构建一个Web页面以表格形式显示已结账的订单信息中每个商品的名称和订单总额,您可以按照以下步骤进行操作:
1. 创建一个HTML页面,可以使用以下代码作为基础:
```html
<!DOCTYPE html>
<html>
<head>
<title>已结账订单信息</title>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
border: 1px solid black;
padding: 8px;
text-align: left;
}
th {
background-color: #ddd;
}
</style>
</head>
<body>
<h1>已结账订单信息</h1>
<table id="orderTable">
<tr>
<th>商品名称</th>
<th>订单总额</th>
</tr>
</table>
<script src="script.js"></script>
</body>
</html>
```
2. 创建一个名为`script.js`的JavaScript文件,用于从MongoDB中获取已结账订单信息,并将其填充到表格中。以下是一个示例代码:
```javascript
// 获取表格元素
const orderTable = document.getElementById('orderTable');
// 使用fetch从后端获取已结账订单信息
fetch('/paidOrders')
.then(response => response.json())
.then(data => {
// 遍历订单数据并将其添加到表格中
data.forEach(order => {
const row = orderTable.insertRow();
const nameCell = row.insertCell();
const totalCell = row.insertCell();
// 填充商品名称和订单总额
nameCell.textContent = order.productName;
totalCell.textContent = order.total;
});
})
.catch(error => console.error(error));
```
3. 创建一个后端API来提供已结账订单信息。您可以使用任何后端技术(如Node.js、Python Flask等)来实现此API,并从MongoDB中检索已结账订单数据并将其返回给前端。以下是一个示例代码(使用Node.js和Express):
```javascript
const express = require('express');
const app = express();
// 假设您已经设置了MongoDB连接和集合
const MongoDBCollection = ...;
// 定义路由,用于获取已结账订单信息
app.get('/paidOrders', (req, res) => {
// 从MongoDB中获取已结账订单数据
MongoDBCollection.find({ paid: true })
.toArray((err, orders) => {
if (err) {
console.error(err);
res.status(500).send('Internal Server Error');
} else {
// 返回订单数据
res.json(orders);
}
});
});
// 启动服务器
app.listen(3000, () => {
console.log('Server started on port 3000');
});
```
请确保您已经安装了所需的依赖项(如express和mongodb驱动程序),并将代码中的MongoDB连接和集合替换为您自己的实际值。
最后,在您的Web服务器上部署HTML页面、JavaScript文件和后端API,并确保MongoDB服务器也在运行。访问页面时,它将从MongoDB中获取已结账订单信息并以表格形式显示在页面上。
阅读全文
相关推荐
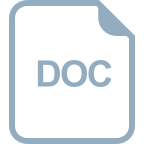
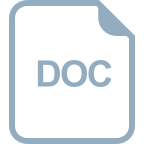
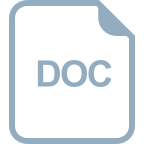
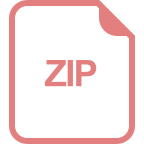
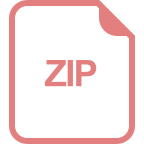
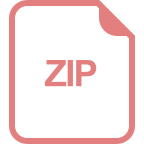
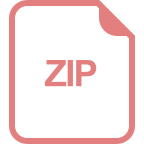
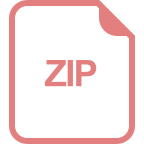
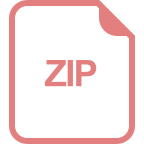
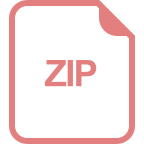
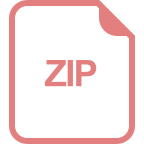
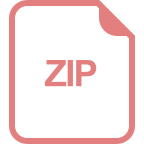
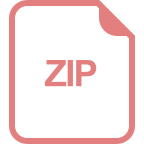
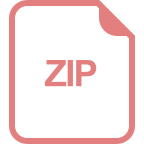
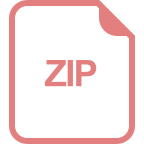
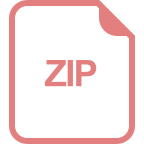
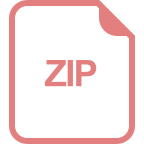
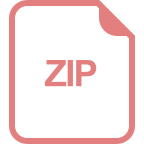
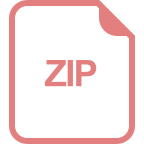